Problem Statement:
- 44,158 cases of motor vehicle theft were reported in 2018; only 19.6% of cases were solved.
- More than four vehicles are stolen every hour in the national capital with motorcycles being the prime target, according to statistics shared by the Delhi Police.
- According to a senior police officer, motorcycles are used frequently for street crimes like snatching and robberies.
Solution:
- If we can control means ON & OFF the bike anywhere & get the exact location of the bike. So we can secure our bike from theft.
- Every bike has an Ignition coil for Starting to bike. It is simple if an ignition coil is not connected to the bike's other system, the bike is not getting started. Vice versa if connected, the bike is getting started.
- Here we used Relay for that connection & disconnection of an ignition coil with the bike Existing system.
- The second point we have to control that Relay from anywhere, for that, we have to use the GSM SIM800L module.
Circuit diagram:
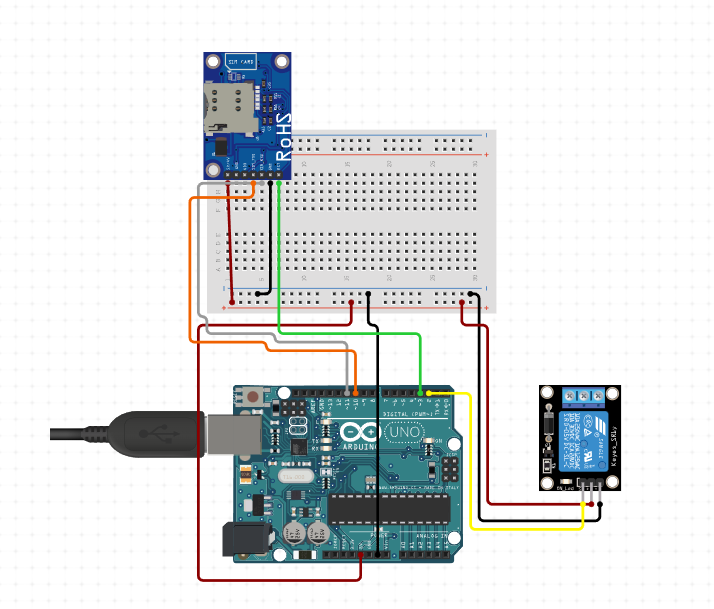
- The relay is connected with 2 GPIO of the Arduino.
- GSM RX pin is connected with 10 GPIO of the Arduino.
- GSM TX pin is connected with 11 GPIO of the Arduino.
- VCC & GND of both Relay and GSM pin are connected with 5v & GND pin of the Arduino.
Relay control through SMS & Getting Location through Simply one call
Code:
#include <SoftwareSerial.h> //Software Serial header to communicate with GSM module
int Relay = 2;
SoftwareSerial SIM800(10, 11); // RX, TX
String Link = "The current Location is https://www.google.com/maps/place/"; //we will append the Lattitude and longitude value later in the program
String responce = "";
String Longitude = "";
String Latitude = "";
String nitesh = "";
String SIM800_send(String incoming) //Function to communicate with SIM800 module
{
SIM800.println(incoming); delay(100); //Print what is being sent to GSM module
String result = "";
while (SIM800.available()) //Wait for result
{
char letter = SIM800.read();
result = result + String(letter); //combine char to string to get result
}
return result; //return the result
}
void setup() {
pinMode(Relay, OUTPUT);
digitalWrite(Relay, HIGH);
Serial.begin(9600); //Serial COM for debugging
SIM800.begin(9600); //Software serial called SIM800 to speak with SIM800 Module
delay(1000); //wait for serial COM to get ready
responce = SIM800_send("AT+SAPBR=3,1,\"CONTYPE\",\"GPRS\" "); //Activate Bearer profile
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
responce = SIM800_send("AT+SAPBR=3,1,\"APN\",\"RCMNET\" "); //Set VPN options =>'RCMNET' 'www'
Serial.print ("Responce:"); Serial.println(responce);
delay(2000);
responce = SIM800_send("AT+SAPBR=1,1"); //Open bearer Profile
Serial.print ("Responce:"); Serial.println(responce); //Open bearer Profile
delay(2000);
responce = SIM800_send("AT+SAPBR=2,1"); //Get the IP address of the bearer profile
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
responce = SIM800_send("AT+CGATT=1"); //Set the SIM800 in GPRS mode
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
responce = SIM800_send("ATE1"); //Enable Echo if not enabled by default
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
responce = SIM800_send("AT+CNMI=2,2,0,0,0"); //Set the SIM800 in GPRS mode
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
}
void prepare_message()
{
//Sample Output for AT+CIPGSMLOC=1,1 ==> +CIPGSMLOC:0,75.802460,26.848892,2019/04/23,08:32:35
//where 26.8488832 is Lattitude and 75.802460 is longitute
int first_comma = responce.indexOf(','); //Find the position of 1st comma
int second_comma = responce.indexOf(',', first_comma+1); //Find the position of 2nd comma
int third_comma = responce.indexOf(',', second_comma+1); //Find the position of 3rd comma
for(int i=first_comma+1; i<second_comma; i++) //Values form 1st comma to 2nd comma is Longitude
Longitude = Longitude + responce.charAt(i);
for(int i=second_comma+1; i<third_comma; i++) //Values form 2nd comma to 3rd comma is Latitude
Latitude = Latitude + responce.charAt(i);
Serial.println(Latitude); Serial.println(Longitude);
nitesh = Link + Latitude + "," + Longitude; //Update the Link with latitude and Logitude values
Serial.println(nitesh);
}
String incoming = "";
void loop() {
if (SIM800.available()) { //Check if the SIM800 Module is telling anything
char a = SIM800.read();
Serial.write(a); //print what the module tells on serial monitor
incoming = incoming + String(a);
if (a == 13) //check for new line
incoming =""; //clear the string if new line is detected
incoming.trim(); //Remove /n or /r from the incomind data
if (incoming=="RING") //If an incoming call is detected the SIM800 module will say "RING" check for it
{
Serial.println ("Sending sms"); delay(1000);
responce = SIM800_send("ATH"); //Hand up the incoming call using ATH
delay (1000);
responce = SIM800_send("ATE0"); //Disable Echo
delay (1000);
responce = ""; Latitude=""; Longitude=""; //initialise all string to null
SIM800.println("AT+CIPGSMLOC=1,1"); delay(5000); //Request for location data
while (SIM800.available())
{
char letter = SIM800.read();
responce = responce + String(letter); //Store the location information in string responce
}
Serial.print("Result Obtained as:"); Serial.print(responce); Serial.println("*******");
prepare_message(); delay(1000); //use prepare_message funtion to prepare the link with the obtained LAT and LONG co-ordinates
SIM800.println("AT+CMGF=1"); //Set the module in SMS mode
delay(1000);
SIM800.println("AT+CMGS=\"+9198XXXXXXXX\""); //Send SMS to this number
delay(1000);
SIM800.println(nitesh); // we have send the string in variable Link
delay(1000);
SIM800.println((char)26);// ASCII code of CTRL+Z - used to terminate the text message
delay(1000);
}
if (a=='b')
{
delay(10);
a=SIM800.read();
if (a=='0')
{
digitalWrite(Relay, LOW);
Serial.println("Relay OFF");
}
else if (a=='1')
{
Serial.println("Relay ON");
digitalWrite(Relay, HIGH);
}
delay(100);
SIM800.println("AT+CMGD=1,4"); // delete all SMS
delay(2000);
}
}
if (Serial.available()) { //For debugging
SIM800.write(Serial.read());
}
}
Explanation of Code:
- Installed <SoftwareSerial.h> library to communicate with the GSM module
#include <SoftwareSerial.h> //Software Serial header to communicate with GSM module
- Define Relay pin & Connection of RX TX pin with Arduino pins
int Relay = 2;
SoftwareSerial SIM800(10, 11); // RX, TX
- AT commands for GSM module
responce = SIM800_send("AT+SAPBR=3,1,\"CONTYPE\",\"GPRS\" "); //Activate Bearer profile
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
responce = SIM800_send("AT+SAPBR=3,1,\"APN\",\"RCMNET\" "); //Set VPN options =>'RCMNET' 'www'
Serial.print ("Responce:"); Serial.println(responce);
delay(2000);
responce = SIM800_send("AT+SAPBR=1,1"); //Open bearer Profile
Serial.print ("Responce:"); Serial.println(responce); //Open bearer Profile
delay(2000);
responce = SIM800_send("AT+SAPBR=2,1"); //Get the IP address of the bearer profile
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
responce = SIM800_send("AT+CGATT=1"); //Set the SIM800 in GPRS mode
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
responce = SIM800_send("ATE1"); //Enable Echo if not enabled by default
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
responce = SIM800_send("AT+CNMI=2,2,0,0,0"); //Set the SIM800 in GPRS mode
Serial.print ("Responce:"); Serial.println(responce);
delay(1000);
- For location we have to prepare a location google map link. First, we define all strings
String Link = "The current Location is https://www.google.com/maps/place/"; //we will append the Lattitude and longitude value later in the program
String responce = "";
String Longitude = "";
String Latitude = "";
String nitesh = "";
- Prepare Message
void prepare_message()
{
//Sample Output for AT+CIPGSMLOC=1,1 ==> +CIPGSMLOC:0,75.802460,26.848892,2019/04/23,08:32:35
//where 26.8488832 is Lattitude and 75.802460 is longitute
int first_comma = responce.indexOf(','); //Find the position of 1st comma
int second_comma = responce.indexOf(',', first_comma+1); //Find the position of 2nd comma
int third_comma = responce.indexOf(',', second_comma+1); //Find the position of 3rd comma
for(int i=first_comma+1; i<second_comma; i++) //Values form 1st comma to 2nd comma is Longitude
Longitude = Longitude + responce.charAt(i);
for(int i=second_comma+1; i<third_comma; i++) //Values form 2nd comma to 3rd comma is Latitude
Latitude = Latitude + responce.charAt(i);
Serial.println(Latitude); Serial.println(Longitude);
nitesh = Link + Latitude + "," + Longitude; //Update the Link with latitude and Logitude values
Serial.println(nitesh);
}
- you can see here nitesh is actual location link which sends in SMS
nitesh = Link + Latitude + "," + Longitude; //Update the Link with latitude and Logitude values
Serial.println(nitesh);
- Condition if we received a call on a GSM SIM card, it automatically cut the call & sends the location link on the register number.
- ATH is AT commands for hang-up calls.
- AT+CMGF=1 is AT command for setting the module in SMS mode.
- AT+CMGS=\"+9198XXXXXXXX\ is AT command for sending SMS on that mobile number.
if (incoming=="RING") //If an incoming call is detected the SIM800 module will say "RING" check for it
{
Serial.println ("Sending sms"); delay(1000);
responce = SIM800_send("ATH"); //Hand up the incoming call using ATH
delay (1000);
responce = SIM800_send("ATE0"); //Disable Echo
delay (1000);
responce = ""; Latitude=""; Longitude=""; //initialise all string to null
SIM800.println("AT+CIPGSMLOC=1,1"); delay(5000); //Request for location data
while (SIM800.available())
{
char letter = SIM800.read();
responce = responce + String(letter); //Store the location information in string responce
}
Serial.print("Result Obtained as:"); Serial.print(responce); Serial.println("*******");
prepare_message(); delay(1000); //use prepare_message funtion to prepare the link with the obtained LAT and LONG co-ordinates
SIM800.println("AT+CMGF=1"); //Set the module in SMS mode
delay(1000);
SIM800.println("AT+CMGS=\"+9198XXXXXXXX\""); //Send SMS to this number
delay(1000);
SIM800.println(nitesh); // we have send the string in variable Link
delay(1000);
SIM800.println((char)26);// ASCII code of CTRL+Z - used to terminate the text message
delay(1000);
}
- Controlling Relay through SMS, here we put condition if SMS received on GSM SIM card "b0" Relay automatically turn OFF. same if received "b1" Relay turns ON. which means if the "b0" SMS received ignition coil is disconnected with other bike systems & connected for "b1".
- AT+CMGD=1,4 is AT commands for Delete all SMS.
if (a=='b')
{
delay(10);
a=SIM800.read();
if (a=='0')
{
digitalWrite(Relay, LOW);
Serial.println("Relay OFF");
}
else if (a=='1')
{
Serial.println("Relay ON");
digitalWrite(Relay, HIGH);
}
delay(100);
SIM800.println("AT+CMGD=1,4"); // delete all SMS
delay(2000);
}
}
if (Serial.available()) { //For debugging
SIM800.write(Serial.read());
}
}
Video
This system is installed in Actual Bike, you check how it's working.