Overview of DHT11
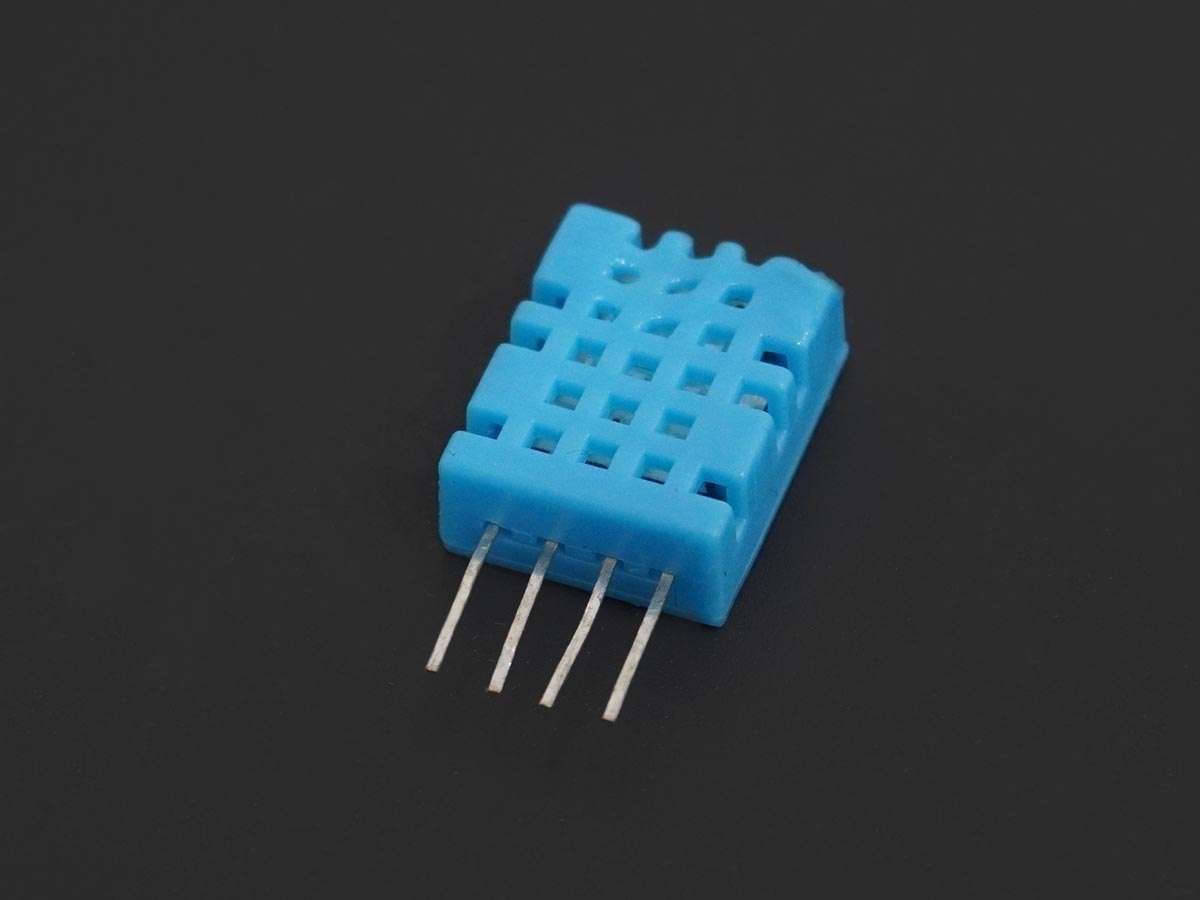
DHT11 is a single wire digital humidity and temperature sensor, which provides humidity and temperature values serially.
It can measure the relative humidity in percentage (20 to 90% RH) and temperature in degree Celsius in the range of 0 to 50°C.
It has 4 pins of which 2 pins are used for supply, 1 is not used and the last one is used for data.
The data is the only pin used for communication. Pulses of different TON and TOFF are decoded as logic 1 or logic 0 or start pulse or end of the frame.
For more information about the DHT11 sensor and how to use it, refer to the topic DHT11 sensor in the sensors and modules topic.
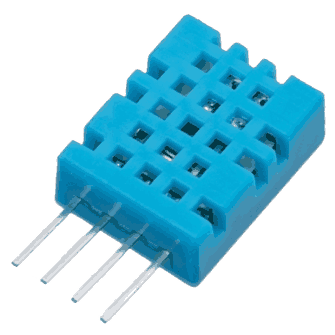
Connection Diagram of DHT11 with ATmega16/32
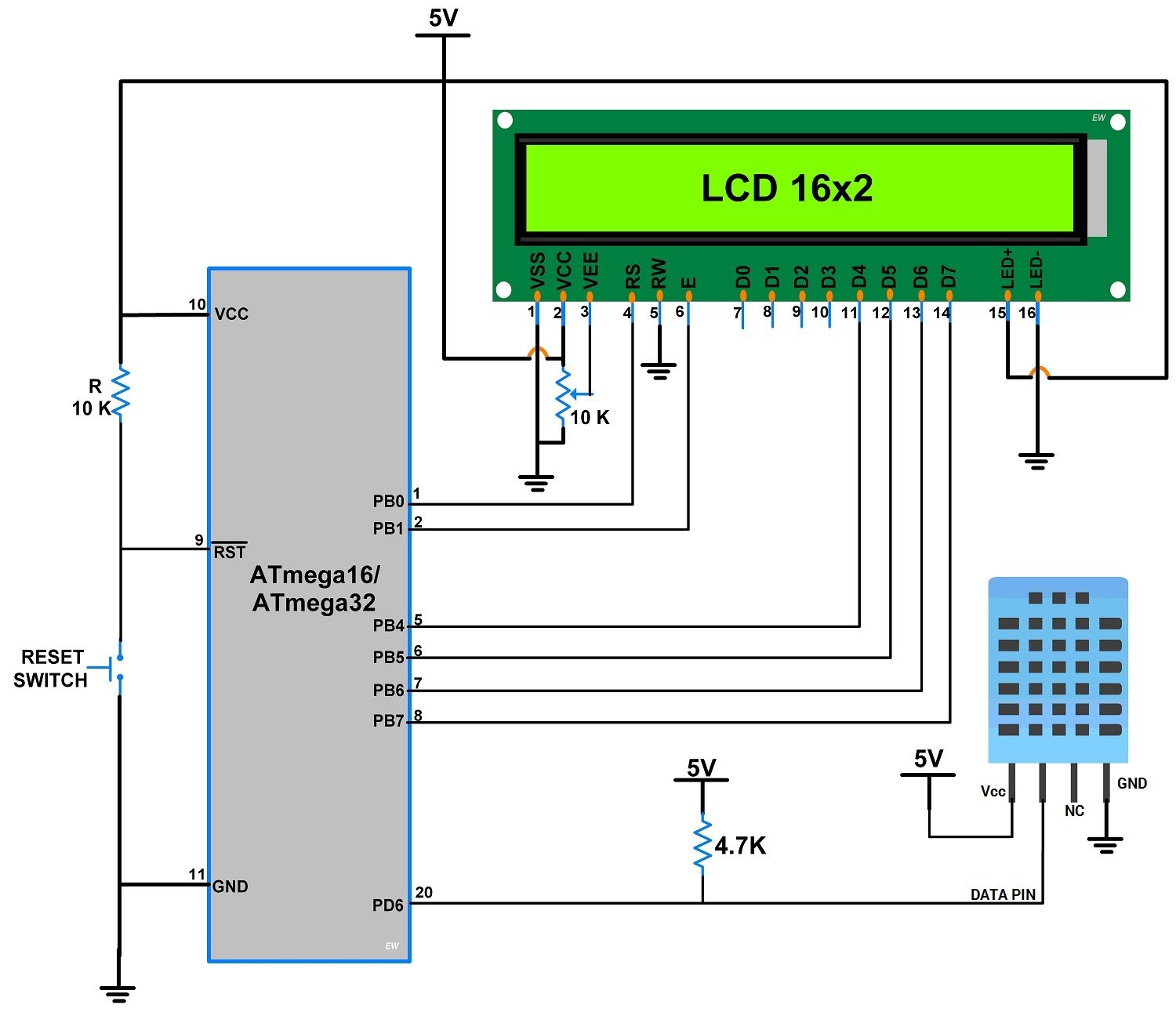
- The above circuit diagram shows the interfacing of AVR ATmega16/ATmega32 to the DHT11 sensor.
In that, LCD is interfaced to PORTB in 4-bit mode, and a DHT11 sensor is connected to PD6 (Pin no 20).
DHT11 Code for ATmega16/32
- First, initialize the LCD16x2_4bit library.
- Define pin no. to interface DHT11 sensor, in our program we define PD6 (Pin no. 20).
- Send the start pulse to the DHT11 sensor, making low to high.
- Receive the response pulse from the DHT11 sensor.
- After receiving the response, receive 40-bit data serially from the DHT11 sensor.
- Display this received data on LCD16x2 along with error indication.
Code
/*
* ATmega16_DHT11_Project_File.c
*
* http://www.electronicwings.com
*/
#include <avr/io.h>
#include <stdlib.h>
#include <stdio.h>
#include "LCD16x2_4bit.h"
#define DHT11_PIN 6
uint8_t c=0,I_RH,D_RH,I_Temp,D_Temp,CheckSum;
void Request() /* Microcontroller send start pulse/request */
{
DDRD |= (1<<DHT11_PIN);
PORTD &= ~(1<<DHT11_PIN); /* set to low pin */
_delay_ms(20); /* wait for 20ms */
PORTD |= (1<<DHT11_PIN); /* set to high pin */
}
void Response() /* receive response from DHT11 */
{
DDRD &= ~(1<<DHT11_PIN);
while(PIND & (1<<DHT11_PIN));
while((PIND & (1<<DHT11_PIN))==0);
while(PIND & (1<<DHT11_PIN));
}
uint8_t Receive_data() /* receive data */
{
for (int q=0; q<8; q++)
{
while((PIND & (1<<DHT11_PIN)) == 0); /* check received bit 0 or 1 */
_delay_us(30);
if(PIND & (1<<DHT11_PIN))/* if high pulse is greater than 30ms */
c = (c<<1)|(0x01); /* then its logic HIGH */
else /* otherwise its logic LOW */
c = (c<<1);
while(PIND & (1<<DHT11_PIN));
}
return c;
}
int main(void)
{
char data[5];
lcdinit(); /* Initialize LCD */
lcd_clear(); /* Clear LCD */
lcd_gotoxy(0,0); /* Enter column and row position */
lcd_print("Humidity =");
lcd_gotoxy(0,1);
lcd_print("Temp = ");
while(1)
{
Request(); /* send start pulse */
Response(); /* receive response */
I_RH=Receive_data(); /* store first eight bit in I_RH */
D_RH=Receive_data(); /* store next eight bit in D_RH */
I_Temp=Receive_data(); /* store next eight bit in I_Temp */
D_Temp=Receive_data(); /* store next eight bit in D_Temp */
CheckSum=Receive_data();/* store next eight bit in CheckSum */
if ((I_RH + D_RH + I_Temp + D_Temp) != CheckSum)
{
lcd_gotoxy(0,0);
lcd_print("Error");
}
else
{
itoa(I_RH,data,10);
lcd_gotoxy(11,0);
lcd_print(data);
lcd_print(".");
itoa(D_RH,data,10);
lcd_print(data);
lcd_print("%");
itoa(I_Temp,data,10);
lcd_gotoxy(6,1);
lcd_print(data);
lcd_print(".");
itoa(D_Temp,data,10);
lcd_print(data);
lcddata(0xDF);
lcd_print("C ");
itoa(CheckSum,data,10);
lcd_print(data);
lcd_print(" ");
}
_delay_ms(10);
}
}
Video of Measuring Temperature and Humidity using DHT11 and Atmega16/32
Components Used |
||
---|---|---|
ATmega 16 ATmega 16 |
X 1 | |
Atmega32 Atmega32 |
X 1 | |
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 | |
LCD16x2 Display LCD16x2 Display |
X 1 | |
Breadboard Breadboard |
X 1 |