Overview of Bluetooth
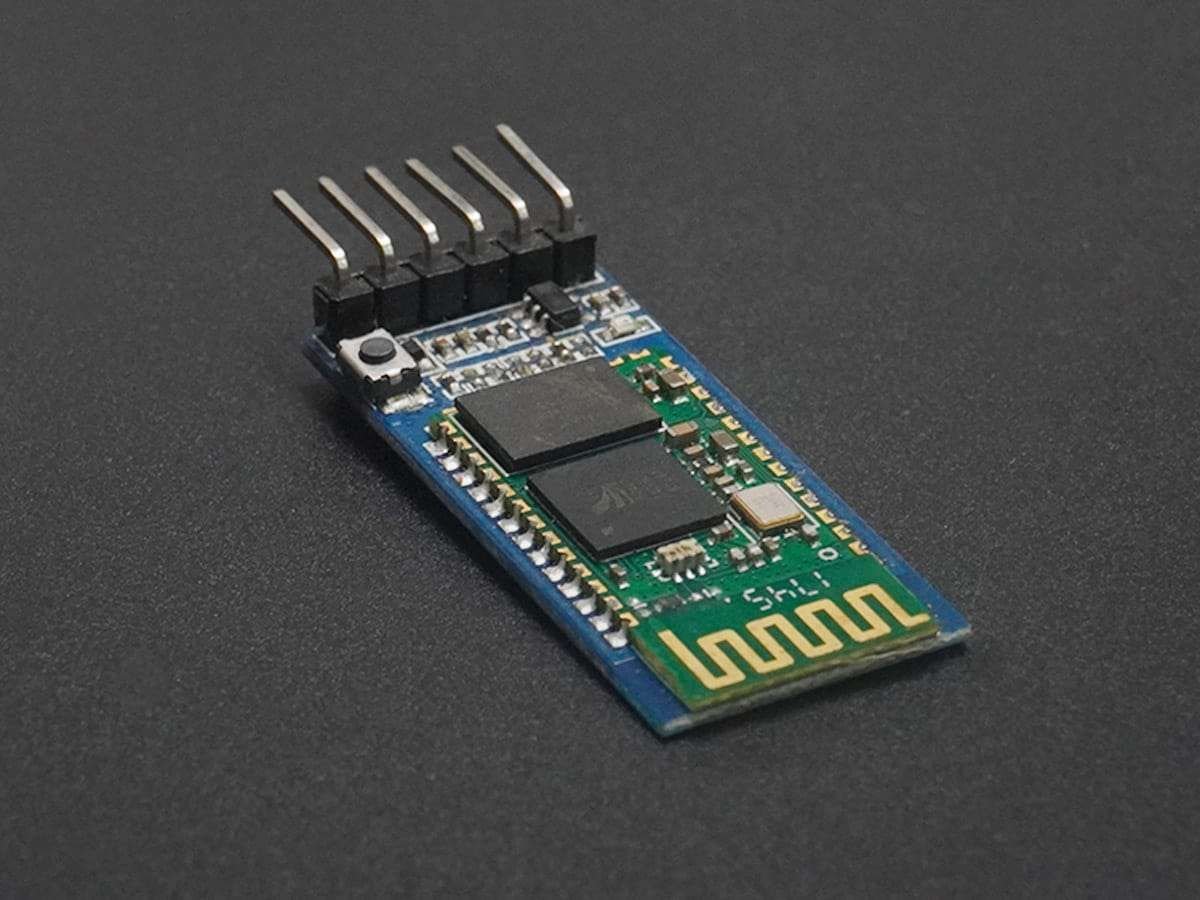
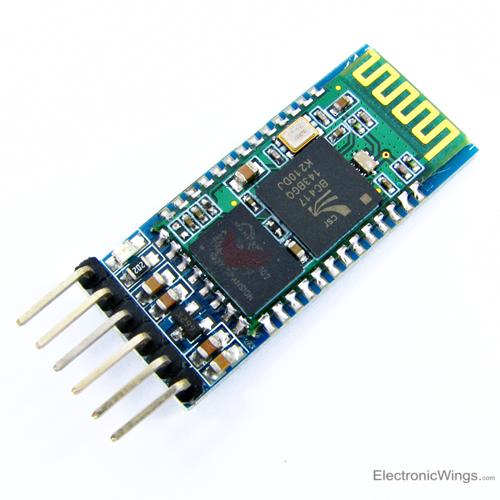
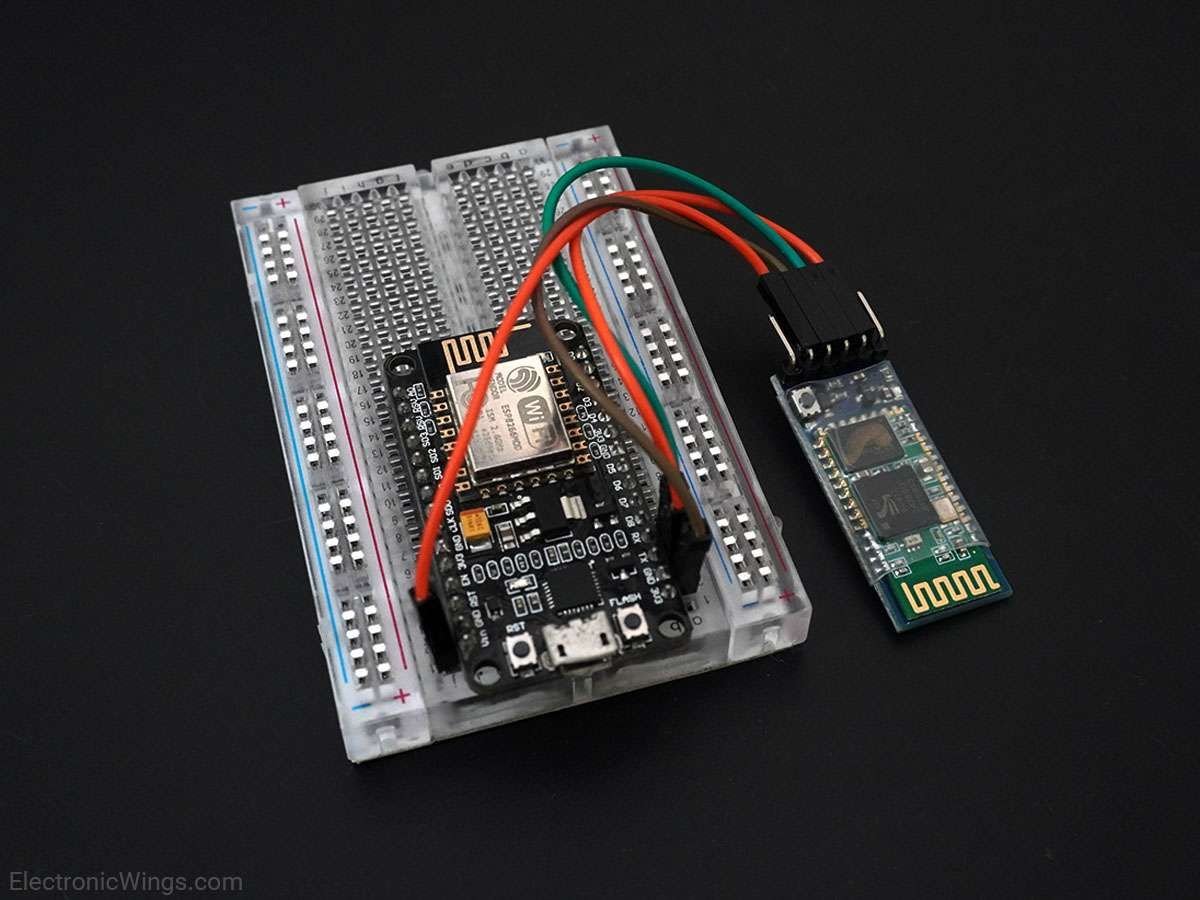
HC-05 is a Bluetooth device used for wireless communication with Bluetooth-enabled devices (like smartphones). It communicates with microcontrollers using serial communication (USART).
This device adds wireless communication protocol to the embedded applications through which it can communicate with any other Bluetooth enabled device.
AT commands are used to command the Bluetooth module. We can change its settings about the password, its name, USART communications settings like baud rate, no. of stop bits or parity, etc.
For more information about the HC-05 Bluetooth module, its pin, and how to use it, refer to the topic HC-05 Bluetooth module in the sensors and modules section.
NodeMCU based ESP8266 has UART serial communication module through which it can communicate Bluetooth module. to know about Lua-based NodeMCU UART functions refer to NodeMCU UART with ESPlorer IDE.
Connection Diagram of HC-05 Bluetooth Module with NodeMCU
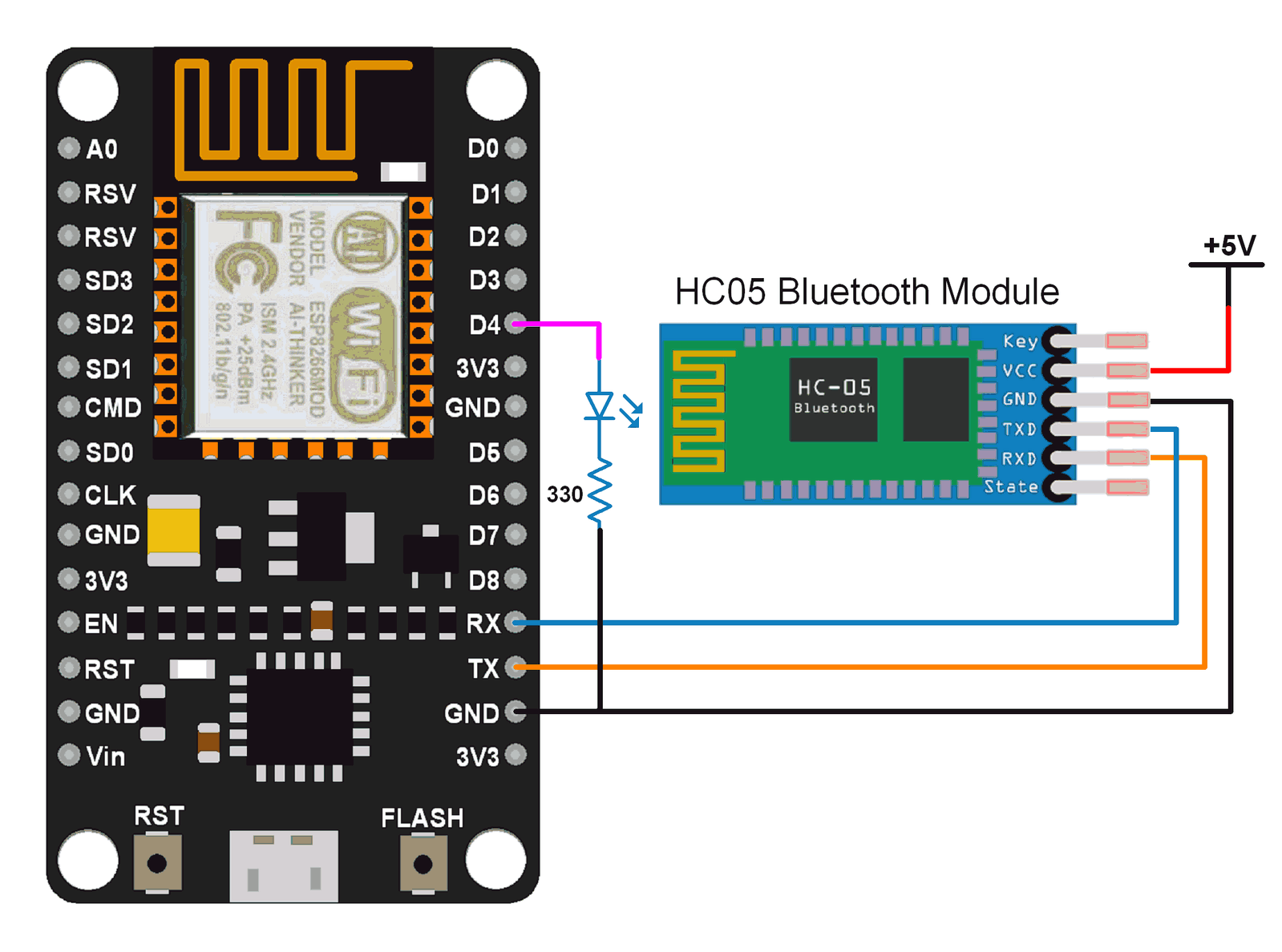
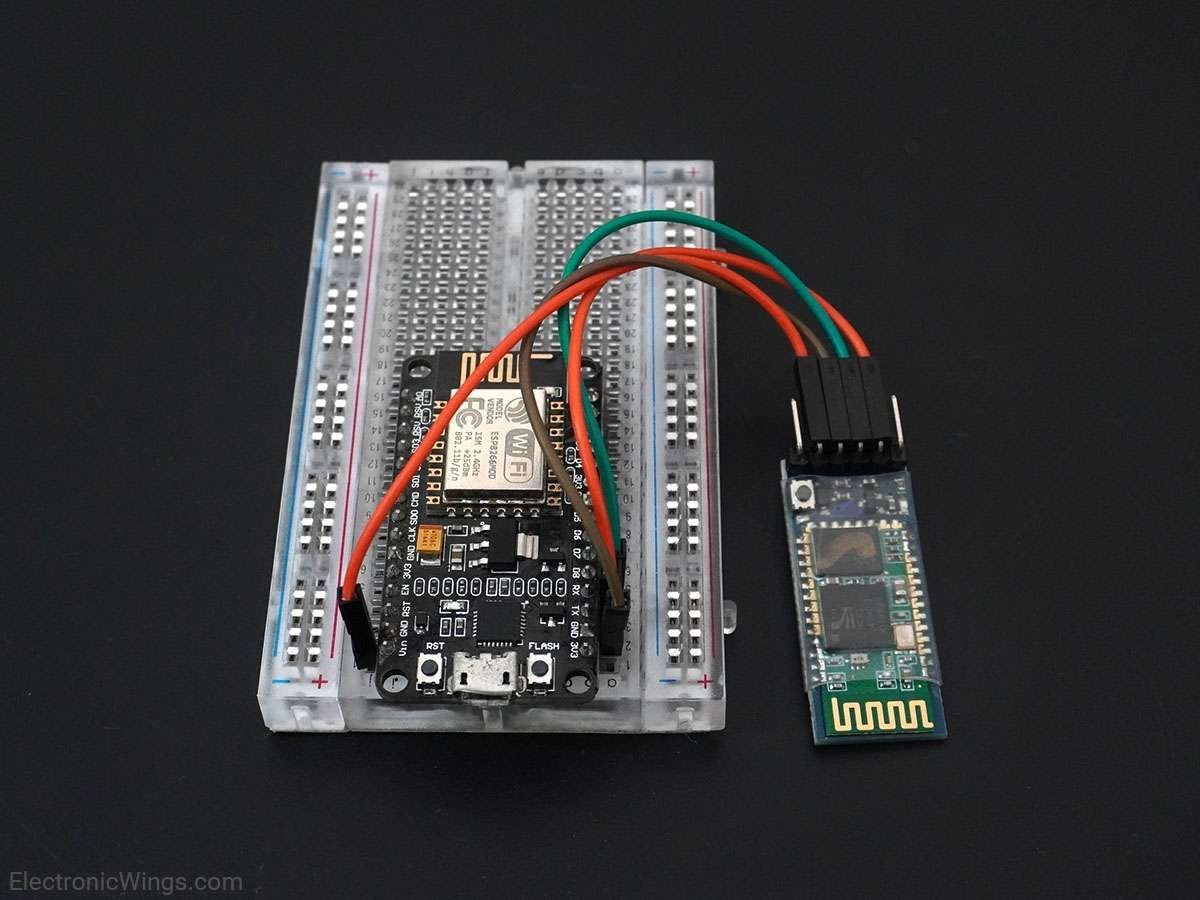
Note: Default Bluetooth name of the device is “HC-05” and the default PIN (password) for connection is either “0000” or “1234”.
Control LED using HC-05 Bluetooth Module using NodeMCU
Let’s develop a small application in which we can control LED ON-OFF through a smartphone.
This is done by interfacing the HC-05 Bluetooth module with NodeMCU. Data from HC-05 is received/ transmitted serially by NodeMCU.
In this application, when 1 is sent from the smartphone, LED will turn ON and if 2 is sent LED will get Turned OFF. If received data is other than 1 or 2, it will return a message to mobile that select the proper option.
We can write codes for NodeMCU DevKit in either Lua Script or C/C++ language. We are using ESPlorer IDE for writing code in Lua scripts and Arduino IDE for writing code in C/C++. To know more refer to Getting started with NodeMCU using ESPlorer IDE (which uses Lua scripting for NodeMCU) and Getting started with NodeMCU using Arduino IDE (which uses C language-based Arduino sketches for NodeMCU).
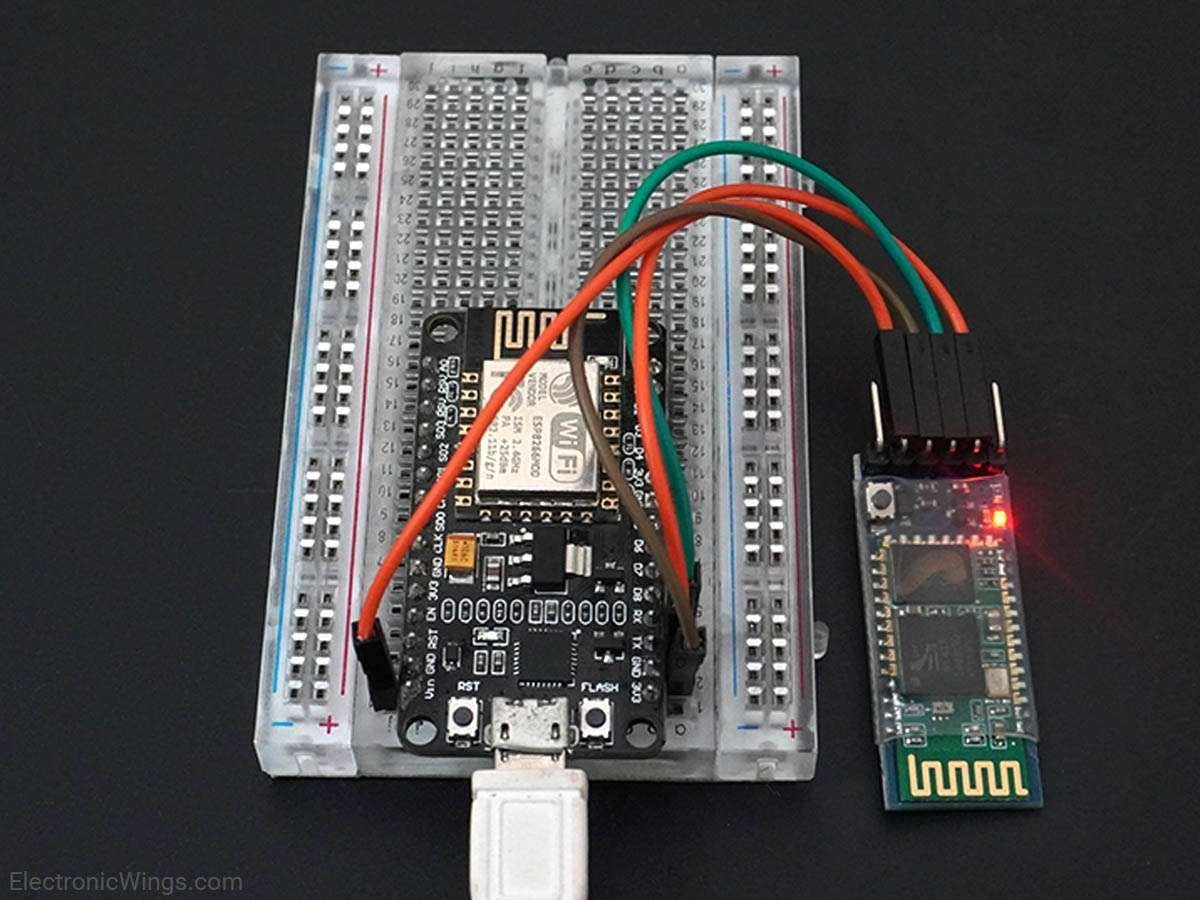
Lua Script for Bluetooth
LEDpin = 4
gpio.mode(LEDpin, gpio.OUTPUT)--set LED pin as output pin
gpio.write(LEDpin, gpio.LOW)-- set LED state initially low
--begin uart with specs
uart.setup(0, 9600, 8, uart.PARITY_NONE, uart.STOPBITS_1, 1)
--set callback function on receive to make decision about LED on/off
uart.on("data",1,
function(data)
if(data == "1") then
gpio.write(LEDpin, gpio.HIGH)
print("LED ON")
elseif(data == "2") then
gpio.write(LEDpin, gpio.LOW)
print("LED OFF")
else
print("select proper option")
end
end, 0)
Below is the response received from NodeMCU Bluetooth while sending commands of the above example on the Bluetooth terminal of the smartphone.
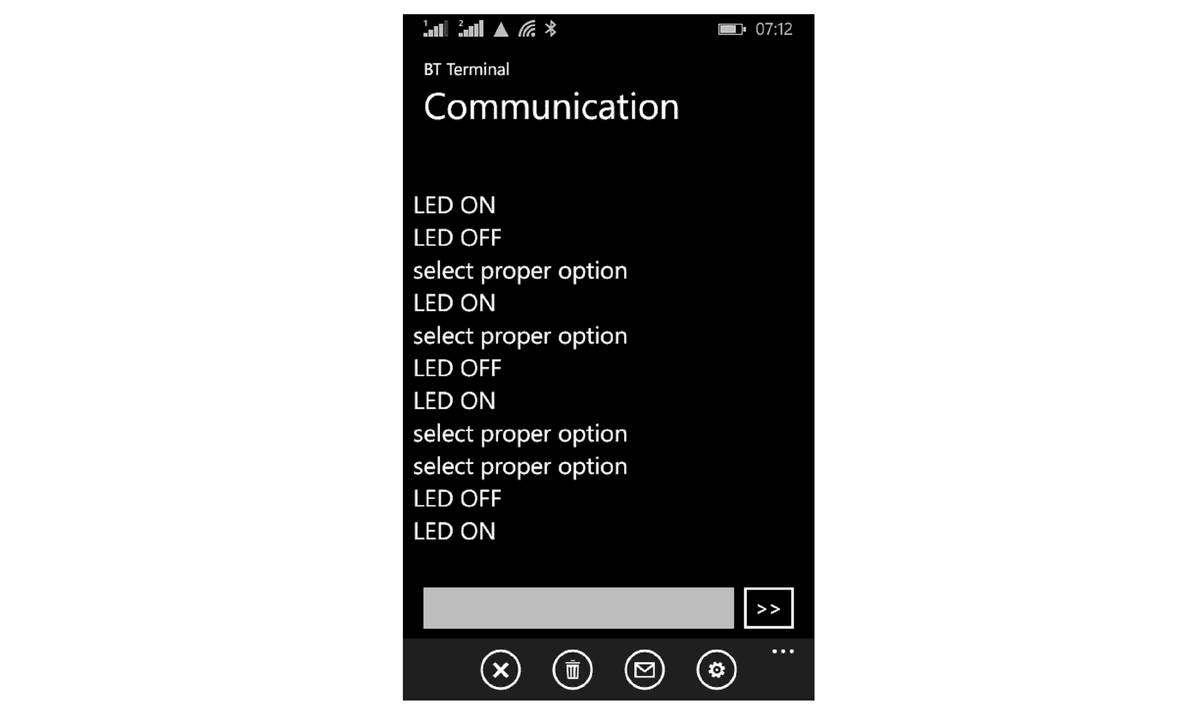
Also, we can write code for the above example from Arduino IDE. To know about how to start using NodeMCU with Arduino IDE refer to Getting started with NodeMCU using Arduino IDE.
HC-05 Bluetooth Code for NodeMCU using Arduino
int LED = D4;
void setup() {
pinMode(LED, OUTPUT);
Serial.begin(9600); /* Define baud rate for serial communication */
}
void loop() {
if (Serial.available()) /* If data is available on serial port */
{
char data_received;
data_received = Serial.read(); /* Data received from bluetooth */
if (data_received == '1')
{
digitalWrite(LED, HIGH);
Serial.write("LED turned ON\n");
}
else if (data_received == '2')
{
digitalWrite(LED, LOW);
Serial.write("LED turned OFF\n");
}
else
{
Serial.write("Select either 1 or 2");
}
}
}
Components Used |
||
---|---|---|
Bluetooth Module HC-05 Bluetooth is a wireless communication protocol used to communicate over short distances. It is used for low power, low cost wireless data transmission applications over 2.4 – 2.485 GHz (unlicensed) frequency band. |
X 1 | |
NodeMCU NodeMCUNodeMCU |
X 1 | |
ESP12F ESP12E |
X 1 | |
LED 5mm LED 5mm |
X 1 |
Downloads |
||
---|---|---|
|
NodeMCU Bluetooth source codes | Download |