Description
- As we know the ESP-NOW is a wireless communication protocol developed by Espressif Systems for its Wi-Fi chips.
- It allows devices to communicate directly with each other without the need for a Wi-Fi access point.
- In this guide, we will send the temperature and humidity data from the master device to many other devices.
- We will be using three ESP8266 boards one is a master ESP8266 (NodeMCU) board and another two ESP8266 (NodeMCU) will become a slave.
- The DHT11 sensor is connected to the master board.
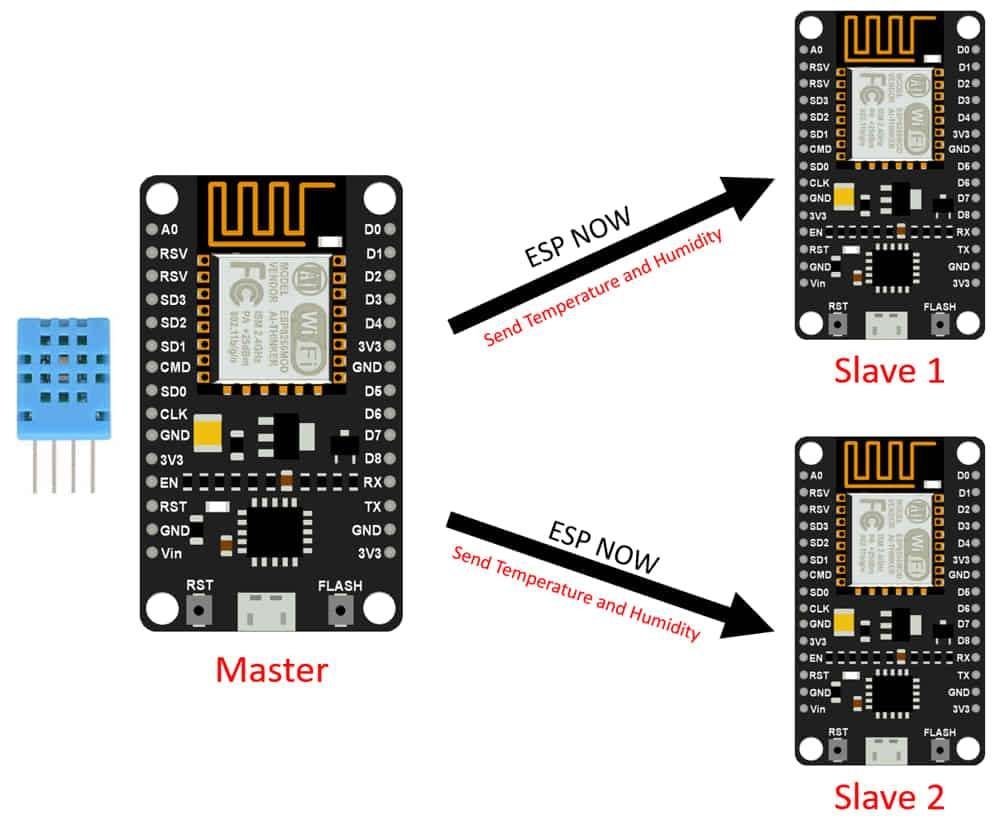
Before going forward we need to know the MAC address of our boards.
So first get the MAC address from the below code and note down it.
Getting Board MAC Address
#include <ESP8266WiFi.h>
void setup(){
Serial.begin(115200);
Serial.println();
Serial.println(WiFi.macAddress());
}
void loop(){}
Output
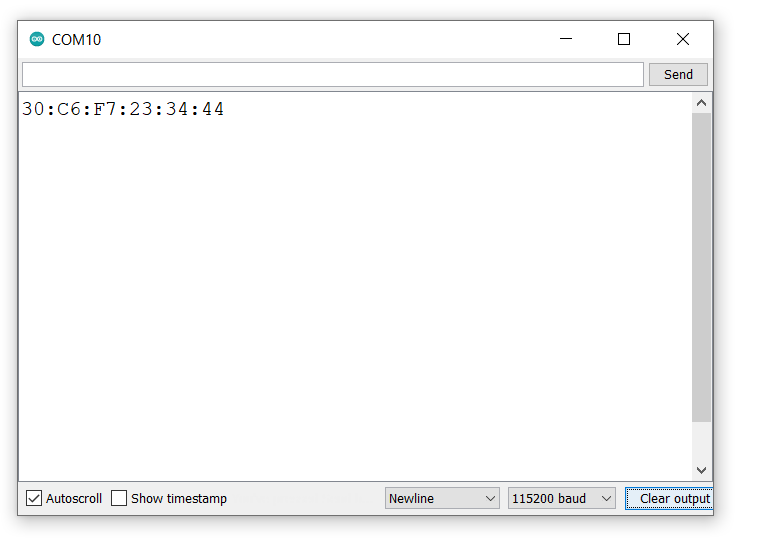
Connection Diagram of DHT11 with ESP8266 (Master Board)
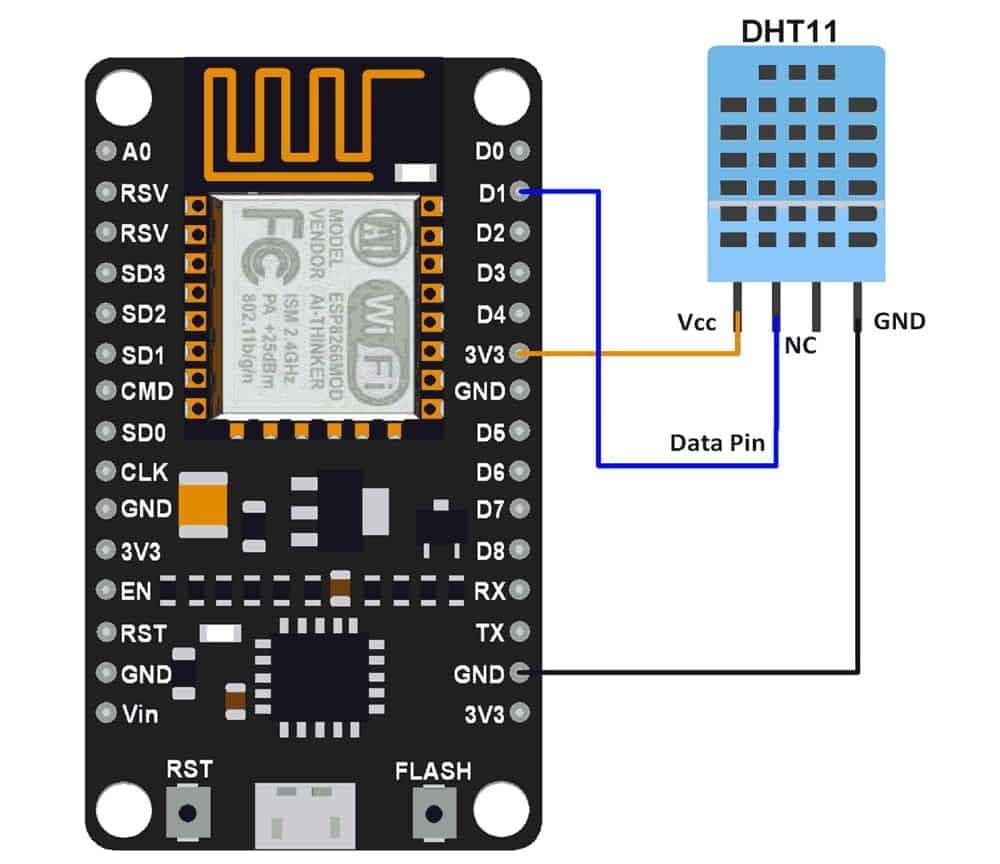
Now copy the below code and upload it on the first esp8266 device. Before uploading you must have to enter the MAC address of your slave device
uint8_t broadcastAddress1[] = {0x30, 0xC6, 0xF7, 0x23, 0x34, 0x44};
uint8_t broadcastAddress2[] = {0x84, 0xF3, 0xEB, 0xCA, 0xF8, 0xD3};
ESP Now DHT11 Sensor Code
#include <ESP8266WiFi.h>
#include <espnow.h>
#include "DHT.h"
DHT dht;
// Enter the MAC address of the first slave device
uint8_t broadcastAddress1[] = {0x84, 0xF3, 0xEB, 0xCA, 0xF8, 0xD3};
// Enter the MAC address of the second slave device
uint8_t broadcastAddress2[] = {0x08, 0x3A, 0x8D, 0xD3, 0x6C, 0xEE};
// Structure to hold DHT11 sensor data
typedef struct struct_message {
float temp;
float hum;
} struct_message;
// Create an instance of the struct_message structure
struct_message DHT11_data;
// Callback function to handle sent data status
void OnDataSent(uint8_t *mac_addr, uint8_t espStatus) {
char macStr[18];
// Convert MAC address to string format
snprintf(macStr, sizeof(macStr), "%02x:%02x:%02x:%02x:%02x:%02x",
mac_addr[0], mac_addr[1], mac_addr[2], mac_addr[3], mac_addr[4], mac_addr[5]);
Serial.print("Delivery Status of: ");
Serial.print(macStr);
Serial.println(espStatus == 0 ? " Sent Successfully" : " Fail to send");
}
void setup() {
Serial.begin(115200); // Initialize the serial monitor
WiFi.mode(WIFI_STA); // Set the WiFi in station mode
dht.setup(D1); // Connect the DHT11's data pin to D1
// Initialize ESP-NOW
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
// Set the role as a controller
esp_now_set_self_role(ESP_NOW_ROLE_CONTROLLER);
// Register callback for data send status
esp_now_register_send_cb(OnDataSent);
// Add peers (slave devices)
esp_now_add_peer(broadcastAddress1, ESP_NOW_ROLE_SLAVE, 1, NULL, 0);
esp_now_add_peer(broadcastAddress2, ESP_NOW_ROLE_SLAVE, 1, NULL, 0);
}
void loop() {
DHT11_data.temp = dht.getTemperature(); // Get the Temperature value
DHT11_data.hum = dht.getHumidity(); // Get the Humidity value
// Send message using ESP-NOW to both slave devices
esp_now_send(0, (uint8_t *) &DHT11_data, sizeof(struct_message));
delay(3000); // Delay for a few seconds before sending the next data
}
- Now upload the code on sender ESP8266.
- After uploading the code open the serial monitor and set the baud rate to 115200
Let’s Understand the code
Add esp_now.h
, DHT.h
, and WiFi.h
libraries.
#include <esp_now.h>
#include <WiFi.h>
#include "DHT.h"
Create the object below
DHT dht;
Now add the MAC address of receiver ESP8266
Our MAC address is 30:C6:F7:23:34:44
uint8_t broadcastAddress1[] = {0x84, 0xF3, 0xEB, 0xCA, 0xF8, 0xD3};
uint8_t broadcastAddress2[] = {0x32, 0xF5, 0xE8, 0x89, 0x45, 0x33};
Here we have created a structure to send the data.
typedef struct struct_message {
float temp;
float hum;
} struct_message;
Create a struct_message
variable called DHT11_data
.
struct_message DHT11_data;
OnDataSent()
is a callback function that will be executed when a message is sent.
void OnDataSent(uint8_t *mac_addr, uint8_t espStatus) {
char macStr[18];
Serial.print("Delivery Status of: ");
snprintf(macStr, sizeof(macStr), "%02x:%02x:%02x:%02x:%02x:%02x",mac_addr[0], mac_addr[1], mac_addr[2], mac_addr[3], mac_addr[4], mac_addr[5]);
Serial.print(macStr);
Serial.println(espStatus == 0 ? " Send Successfully" : " Fail to send");
}
In the setup function,
Initialize the serial monitor
Serial.begin(115200);
set the D1
pin as a data communication Pin.
dht.setup(D1);
Set ESP8266 in a Wi-Fi station Mode:
WiFi.mode(WIFI_STA);
Initialize ESP-NOW:
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
Set the ESP8266 role as a controller, to send data to other devices in the network
esp_now_set_self_role(ESP_NOW_ROLE_CONTROLLER);
Now, register the callback function that will be called when a message is sent.
esp_now_register_send_cb(OnDataSent);
Pair with the slave device to send the data
esp_now_add_peer(broadcastAddress1, ESP_NOW_ROLE_CONTROLLER, 1, NULL, 0);
The arguments for esp_now_add_peer
are:
esp_now_add_peer (mac address, role, wi-fi channel, key, key length)
In the loop function
Get the temperature and humidity reading from DHT11 and store it.
DHT11_data.temp = dht.getTemperature(); /*Get the Temperature value*/
DHT11_data.hum = dht.getHumidity(); /*Get the Humidity value*/
Here we’ll send the temperature and humidity every three seconds using ESPNOW
esp_now_send(0 , (uint8_t *) &msg, sizeof(msg));
0
: Destination sends packet to all MAC addresses recorded by ESP-NOW.
(uint8_t *) &msg
: This is the data you want to send.
sizeof(msg)
: This specifies the size of the data being sent.
Wait for three second
delay(3000);
Now copy the below code and upload it on the second device and check the result on the serial monitor.
Before uploading make sure you have updated your MAC address
uint8_t broadcastAddress[] = {0xXX, 0xXX, 0xXX, 0xXX, 0xXX, 0xXX };
ESP Now ESP8266 NodeMCU Slave-1 Receiver Code
Upload the following code in NodeMCU receiver-1 and check. We will start receiving data.
#include <ESP8266WiFi.h>
#include <espnow.h>
// Variables to store DHT11 sensor data
float DHT11_Temp;
float DHT11_Hum;
// String to hold success status (not used in the code)
String success;
// Define a structure to hold temperature and humidity data
typedef struct struct_message {
float temp;
float hum;
} struct_message;
// Create an instance of the struct_message structure for DHT11 data
struct_message DHT11_data;
// Callback function to handle received data
void OnDataRecv(uint8_t * mac, uint8_t *incomingData, uint8_t len) {
// Copy the received data to the DHT11_data structure
memcpy(&DHT11_data, incomingData, sizeof(DHT11_data));
// Update temperature and humidity values from received data
DHT11_Temp = DHT11_data.temp;
DHT11_Hum = DHT11_data.hum;
// Print temperature and humidity values on the serial monitor
Serial.print("Temperature: ");
Serial.print(DHT11_data.temp);
Serial.println(" ºC");
Serial.print("Humidity: ");
Serial.print(DHT11_data.hum);
Serial.println(" %");
Serial.println();
}
void setup() {
Serial.begin(115200); // Initialize the serial monitor
WiFi.mode(WIFI_STA); // Set the WiFi in station mode
// Initialize ESP-NOW
if (esp_now_init() != 0) {
Serial.println("Error initializing ESP-NOW");
return;
}
// Set the role as a slave
esp_now_set_self_role(ESP_NOW_ROLE_SLAVE);
// Register ESP-NOW receive callback
esp_now_register_recv_cb(OnDataRecv);
}
void loop() {}
- Now upload the code on NodeMCU Receiver.
- After uploading the code open the serial monitor and set the baud rate to 115200
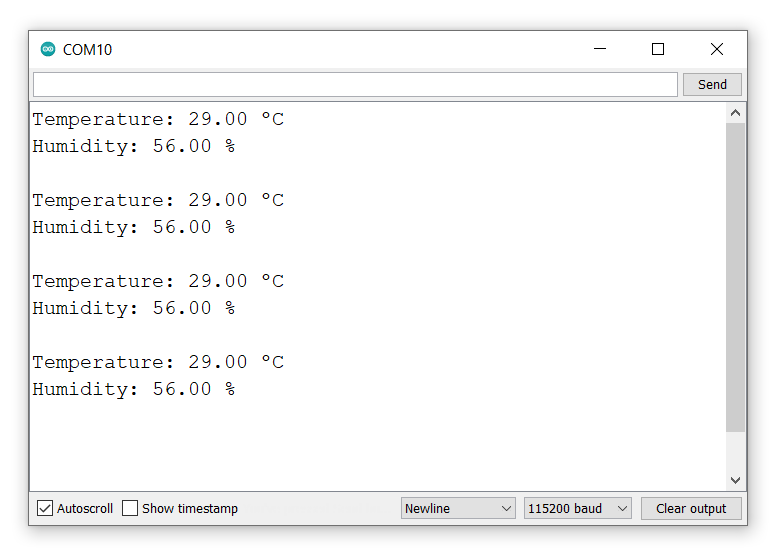
Understand the code
Add esp8266WiFI.h
and espnow.h
libraries.
#include <ESP8266WiFi.h>
#include <espnow.h>
Here we have created a structure to send and receive the data.
typedef struct struct_message {
float temp;
float hum;
} struct_message;
Create a struct_message
variable called DHT11_data
and BMP180_data
.
struct_message DHT11_data;
Create a callback function that will be called when the ESP8266 receives the data via ESP-NOW.
void OnDataRecv(uint8_t * mac, uint8_t *incomingData, int len) {
memcpy(&DHT11_data, incomingData, sizeof(DHT11_data));
DHT11_Temp = DHT11_data.temp;
DHT11_Hum = DHT11_data.temp;
Serial.print("Temperature: ");
Serial.print(DHT11_data.temp);
Serial.println(" ºC");
Serial.print("Humidity: ");
Serial.print(DHT11_data.hum);
Serial.println(" %");
Serial.println();
}
In the setup function,
Initialize the serial monitor
Serial.begin(115200);
Set ESP8266 in a Wi-Fi station Mode:
WiFi.mode(WIFI_STA);
Initialize ESP-NOW:
if (esp_now_init() != ESP_OK) {
Serial.println("Error initializing ESP-NOW");
return;
}
Now, register the callback function that will be called when a message is received.
esp_now_register_recv_cb(OnDataRecv);
Receiving Data in Slave-2
Similarly, as above, upload the same code in receiver-2 and we will receive the same data.
With this, we can conclude that we can send data from one device to multiple devices via ESP-NOW protocol.
Components Used |
||
---|---|---|
NodeMCU NodeMCUNodeMCU |
X 3 | |
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 |