DHT11 Sensor
DHT11 is a single-wire digital humidity and temperature sensor, which provides humidity and temperature values serially.
It can measure the relative humidity in percentage (20 to 90% RH) and temperature in degree Celsius in the range of 0 to 50°C.
It has 4 pins of which 2 pins are used for supply, 1 is not used and the last one is used for data.
The data is the only pin used for communication. Pulses of different TON and TOFF are decoded as logic 1 or logic 0 or start pulse or end of the frame.
For more information about the DHT11 sensor and how to use it, refer to the topic DHT11 sensor in the sensors and modules topic.
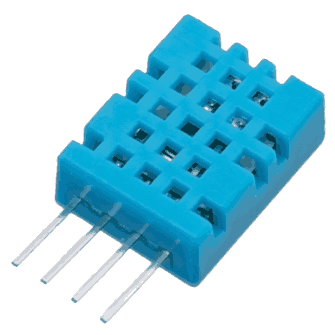
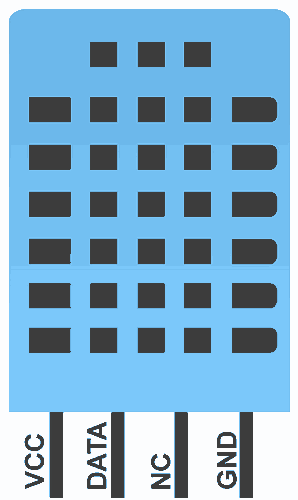
Connection Of DHT11 Sensor with 8051
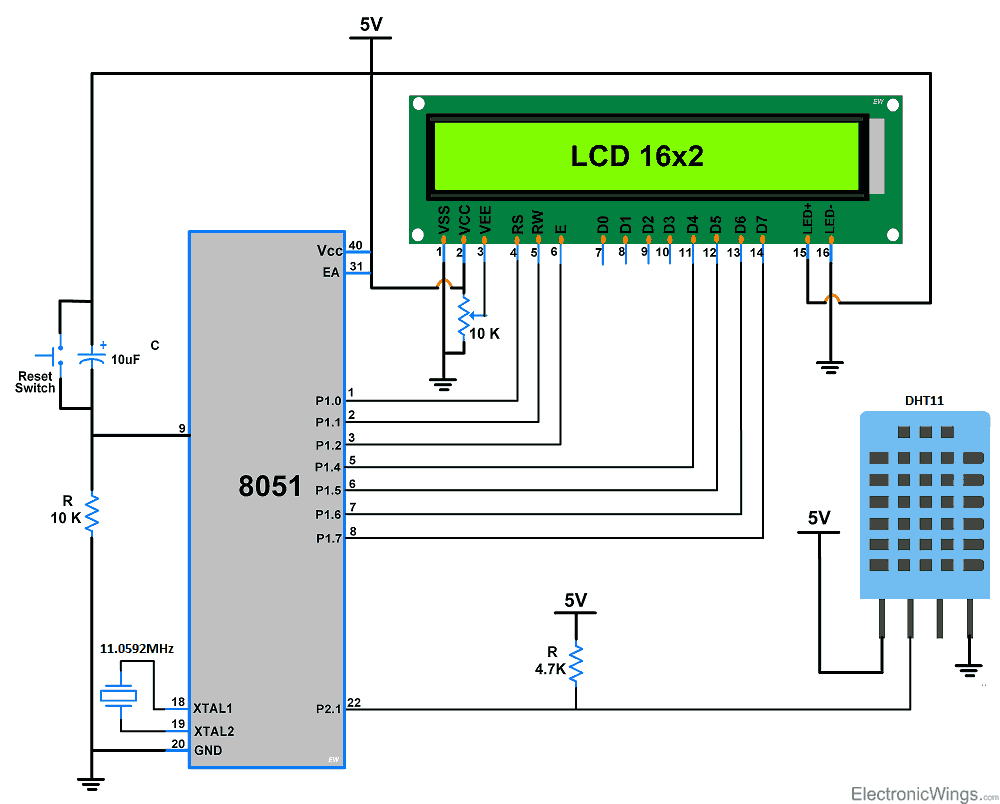
- The above circuit diagram shows the interfacing of 8051 with the DHT11 sensor.
- In that, a DHT11 sensor is connected to P2.1(Pin No 22) of the microcontroller.
DHT11 Sensor 8051 Programming Steps
- First, initialize the LCD16x2_4bit.h library.
- Define pin no. to interface the DHT11 sensor, in our program we define P2.1 (Pin no.22)
- Send the start pulse to the DHT11 sensor by making low to high on the data pin.
- Receive the response pulse from the DHT11 sensor.
- After receiving the response, receive 40-bit data serially from the DHT11 sensor.
- Display this received data on LCD16x2 along with the error indication.
DHT11 Sensor 8051 Code
/*
* DHT11 Interfacing with 8051
* http://www.electronicwings.com
*/
#include<reg51.h>
#include<stdio.h>
#include<string.h>
#include <stdlib.h>
#include "LCD16x2_4bit.h"
sbit DHT11=P2^1; /* Connect DHT11 output Pin to P2.1 Pin */
int I_RH,D_RH,I_Temp,D_Temp,CheckSum;
void timer_delay20ms() /* Timer0 delay function */
{
TMOD = 0x01;
TH0 = 0xB8; /* Load higher 8-bit in TH0 */
TL0 = 0x0C; /* Load lower 8-bit in TL0 */
TR0 = 1; /* Start timer0 */
while(TF0 == 0); /* Wait until timer0 flag set */
TR0 = 0; /* Stop timer0 */
TF0 = 0; /* Clear timer0 flag */
}
void timer_delay30us() /* Timer0 delay function */
{
TMOD = 0x01; /* Timer0 mode1 (16-bit timer mode) */
TH0 = 0xFF; /* Load higher 8-bit in TH0 */
TL0 = 0xF1; /* Load lower 8-bit in TL0 */
TR0 = 1; /* Start timer0 */
while(TF0 == 0); /* Wait until timer0 flag set */
TR0 = 0; /* Stop timer0 */
TF0 = 0; /* Clear timer0 flag */
}
void Request() /* Microcontroller send request */
{
DHT11 = 0; /* set to low pin */
timer_delay20ms(); /* wait for 20ms */
DHT11 = 1; /* set to high pin */
}
void Response() /* Receive response from DHT11 */
{
while(DHT11==1);
while(DHT11==0);
while(DHT11==1);
}
int Receive_data() /* Receive data */
{
int q,c=0;
for (q=0; q<8; q++)
{
while(DHT11==0);/* check received bit 0 or 1 */
timer_delay30us();
if(DHT11 == 1) /* If high pulse is greater than 30ms */
c = (c<<1)|(0x01);/* Then its logic HIGH */
else /* otherwise its logic LOW */
c = (c<<1);
while(DHT11==1);
}
return c;
}
void main()
{
unsigned char dat[20];
LCD_Init(); /* initialize LCD */
while(1)
{
Request(); /* send start pulse */
Response(); /* receive response */
I_RH=Receive_data(); /* store first eight bit in I_RH */
D_RH=Receive_data(); /* store next eight bit in D_RH */
I_Temp=Receive_data(); /* store next eight bit in I_Temp */
D_Temp=Receive_data(); /* store next eight bit in D_Temp */
CheckSum=Receive_data();/* store next eight bit in CheckSum */
if ((I_RH + D_RH + I_Temp + D_Temp) != CheckSum)
{
LCD_String_xy(0,0,"Error");
}
else
{
sprintf(dat,"Hum = %d.%d",I_RH,D_RH);
LCD_String_xy(0,0,dat);
sprintf(dat,"Tem = %d.%d",I_Temp,D_Temp);
LCD_String_xy(1,0,dat);
LCD_Char(0xDF);
LCD_String("C");
memset(dat,0,20);
sprintf(dat,"%d ",CheckSum);
LCD_String_xy(1,13,dat);
}
delay(100);
}
}
Video DHT11 Sensor Output
DHT11 Sensor Display Temperature and Humidity on LCD16x2 using 8051
Components Used |
||
---|---|---|
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 | |
Breadboard Breadboard |
X 1 | |
8051 AT89c51 8051 AT89c51 |
X 1 |