Description
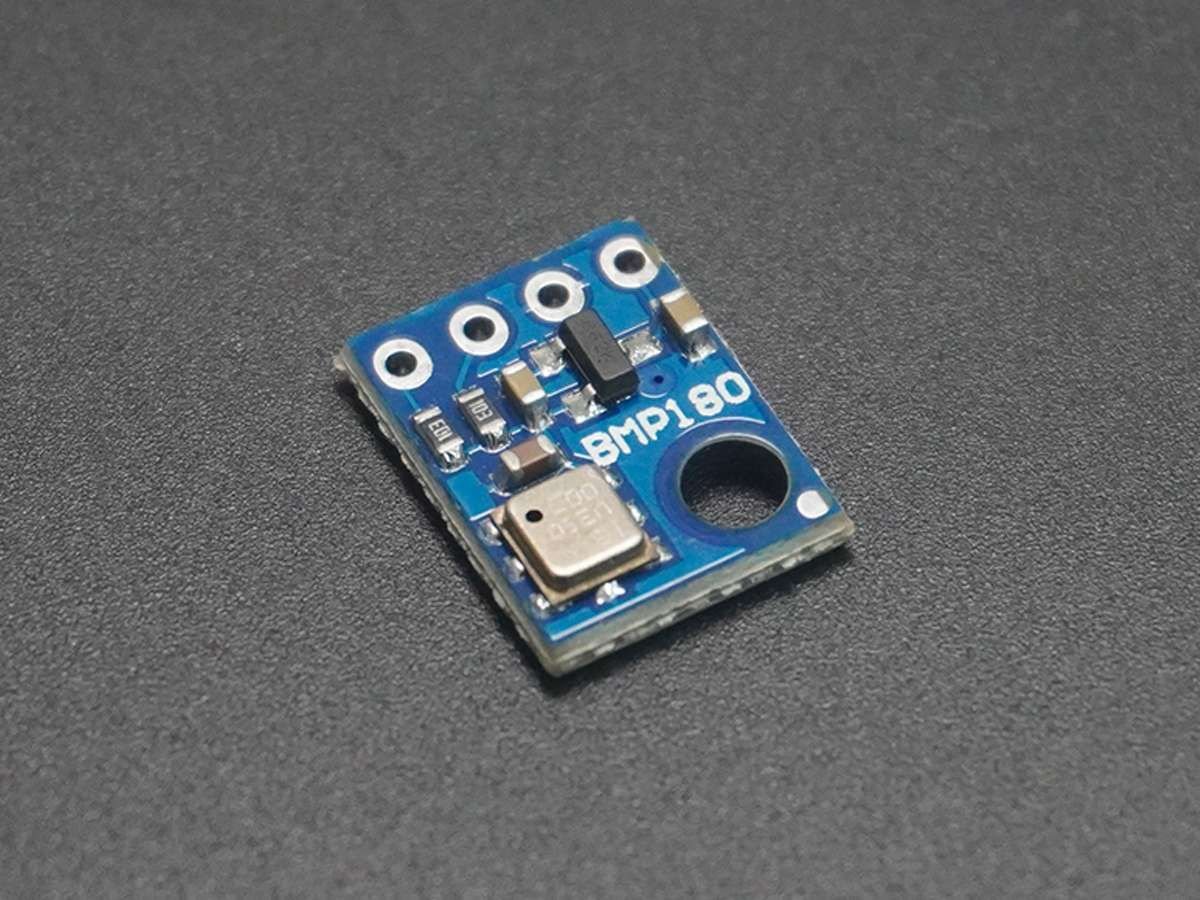
- The BMP180 is a popular digital barometric pressure and temperature sensor developed by Bosch Sensortec.
- It is designed to measure atmospheric pressure, temperature, and altitude with high accuracy and resolution.
- The BMP180 uses a piezo-resistive pressure sensor and a temperature sensor to provide accurate measurements of atmospheric pressure and temperature.
- The pressure sensor measures the absolute pressure of the air, and the temperature sensor compensates for temperature variations.
- The BMP180 then uses these measurements to calculate the altitude above sea level.
- The module uses an I2C communication protocol for communicating with the microcontroller.
BMP180 Module Specification
- Pressure range: 300hPa to 1100hPa and resolution of 0.1hPa.
- Altitude range: -500 m to 9000 m with a resolution of 0.1m (0.328 ft).
- Accuracy: ±1hPa for pressure and ±1°C for temperature and ±1 meter under normal conditions.
- Temperature range: -40°C to +85°C
- Interface: I2C upto 3.4MHz
- Supply voltage: 1.8 V to 3.6 V
BMP180 Pinouts
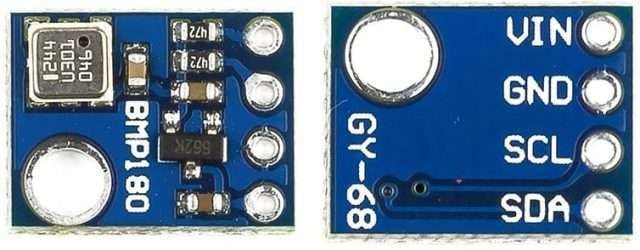
BMP180 Pin Description
- VCC: Connected to the positive supply of 3.3V
- GND: Common Ground pin.
- SCL: Serial Clock Line pin for I2C communication.
- SDA: Serial Data pin for I2C communication.
BMP180 Hardware Connection with Arduino
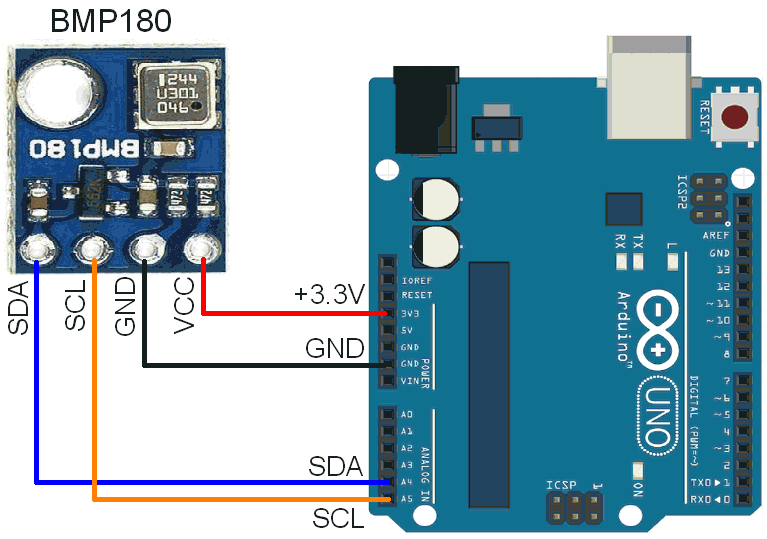
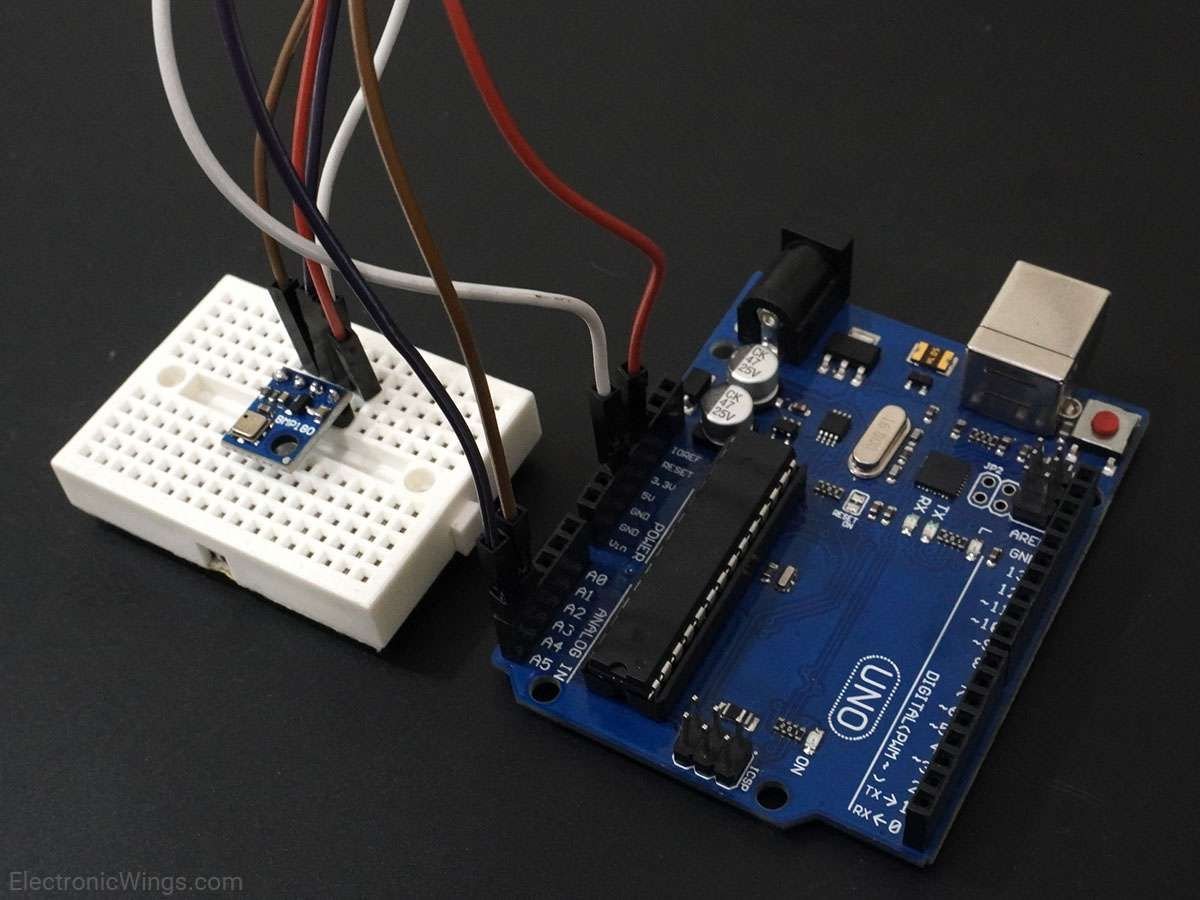
Extracting Data from BMP180 using Arduino
Reading the values from BMP180 and displaying them on the Arduino IDE’s serial monitor.
Here, we'll make use of Adafruit’s BMP180 library named Adafruit_BMP085.h
We will download it from the library manager of Arduino IDE.
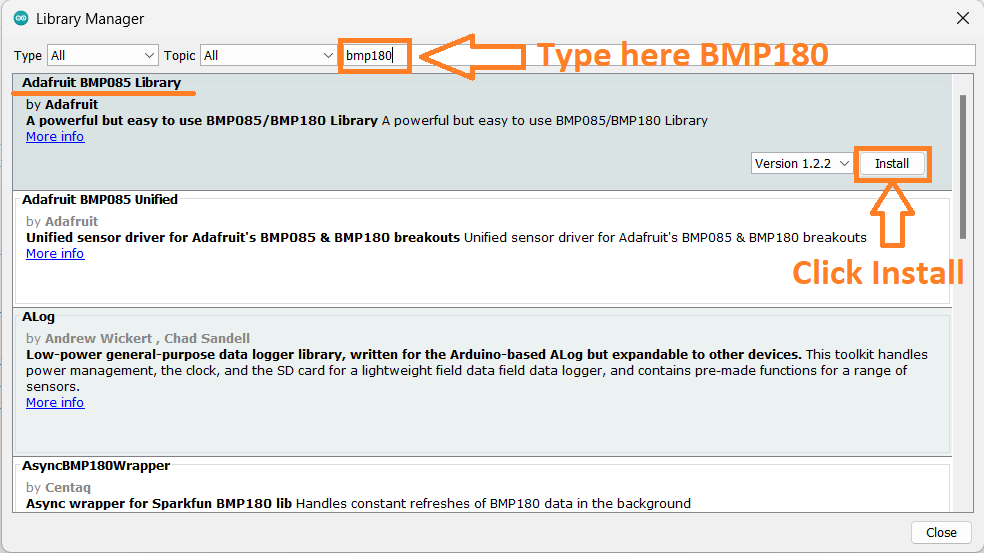
Now open an example of Adafruit ADXL345 io dashboard. To open it navigate to File ->Examples -> Adafruit BMP180 Library-> bmp085test
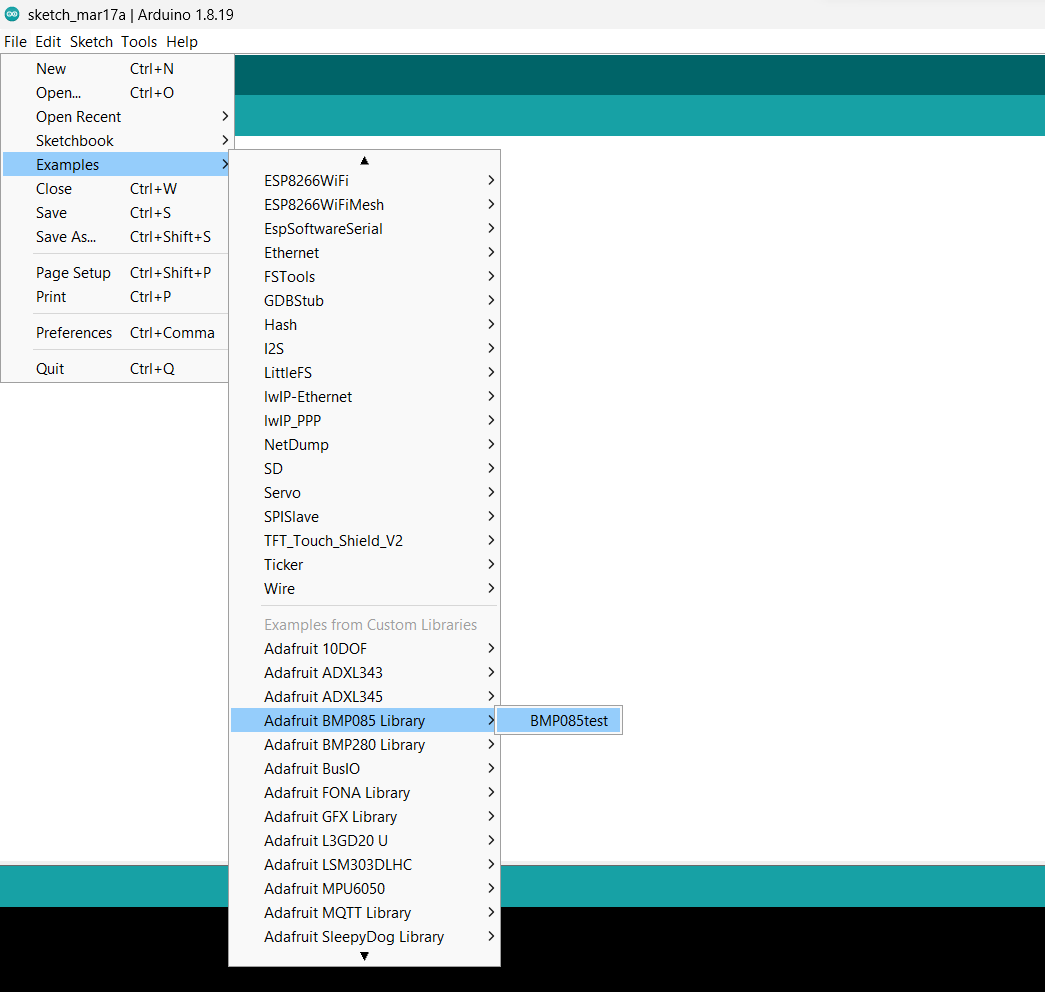
Code for reading values and display it on the Serial Monitor
#include <Adafruit_BMP085.h>
/***************************************************
This is an example for the BMP085 Barometric Pressure & Temp Sensor
Designed specifically to work with the Adafruit BMP085 Breakout
----> https://www.adafruit.com/products/391
These pressure and temperature sensors use I2C to communicate, 2 pins
are required to interface
Adafruit invests time and resources providing this open source code,
please support Adafruit and open-source hardware by purchasing
products from Adafruit!
Written by Limor Fried/Ladyada for Adafruit Industries.
BSD license, all text above must be included in any redistribution
****************************************************/
// Connect VCC of the BMP085 sensor to 3.3V (NOT 5.0V!)
// Connect GND to Ground
// Connect SCL to i2c clock - on '168/'328 Arduino Uno/Duemilanove/etc thats Analog 5
// Connect SDA to i2c data - on '168/'328 Arduino Uno/Duemilanove/etc thats Analog 4
// EOC is not used, it signifies an end of conversion
// XCLR is a reset pin, also not used here
Adafruit_BMP085 bmp;
void setup() {
Serial.begin(9600);
if (!bmp.begin()) {
Serial.println("Could not find a valid BMP085 sensor, check wiring!");
while (1) {}
}
}
void loop() {
Serial.print("Temperature = ");
Serial.print(bmp.readTemperature());
Serial.println(" *C");
Serial.print("Pressure = ");
Serial.print(bmp.readPressure());
Serial.println(" Pa");
// Calculate altitude assuming 'standard' barometric
// pressure of 1013.25 millibar = 101325 Pascal
Serial.print("Altitude = ");
Serial.print(bmp.readAltitude());
Serial.println(" meters");
Serial.print("Pressure at sealevel (calculated) = ");
Serial.print(bmp.readSealevelPressure());
Serial.println(" Pa");
// you can get a more precise measurement of altitude
// if you know the current sea level pressure which will
// vary with weather and such. If it is 1015 millibars
// that is equal to 101500 Pascals.
Serial.print("Real altitude = ");
Serial.print(bmp.readAltitude(101500));
Serial.println(" meters");
Serial.println();
delay(500);
}
- Now upload the code.
- After uploading the code open the serial monitor and set the baud rate to 9600 to see the output.
Output on the serial monitor
.png)
Let’s understand the code
In the beginning, we imported the library of BMP180.
Adafruit_BMP085.h
library has all the functions and classes for extracting the data from the module.
#include <Adafruit_BMP085.h>
Now we created an object for Adafruit_BMP085
class as bmp
Adafruit_BMP085 bmp;
- In the setup function, we have defined the baud rate to 9600.
- Now, we have used an if condition to check if the module is connected or not using
begin()
function.
void setup() {
Serial.begin(9600);
if (!bmp.begin()) {
Serial.println("Could not find a valid BMP085 sensor, check wiring!");
while (1) {}
}
}
- In the loop function, we have extracted the data from the module using functions such as
readTemperature()
,readPressure()
,readAltitude()
, andreadSealevelPressure()
. - The data is extracted using these functions and displayed the values on the serial monitor one by one repeatedly.
Serial.print("Temperature = ");
Serial.print(bmp.readTemperature());
Serial.println(" *C");
Serial.print("Pressure = ");
Serial.print(bmp.readPressure());
Serial.println(" Pa");
Serial.print("Altitude = ");
Serial.print(bmp.readAltitude());
Serial.println(" meters");
Serial.print("Pressure at sealevel (calculated) = ");
Serial.print(bmp.readSealevelPressure());
Serial.println(" Pa");
Serial.print("Real altitude = ");
Serial.print(bmp.readAltitude(101500));
Serial.println(" meters");
Serial.println();
delay(500);
Components Used |
||
---|---|---|
BMP180 Digital Pressure sensor BMP180 is an ultra low power, high precision barometric pressure sensor with I2C serial interface. |
X 1 | |
Arduino UNO Arduino UNO |
X 1 |
Downloads |
||
---|---|---|
|
BMP180_Arduino_SerialMonitor | Download |