Overview of MAX7219 7-Segment Display
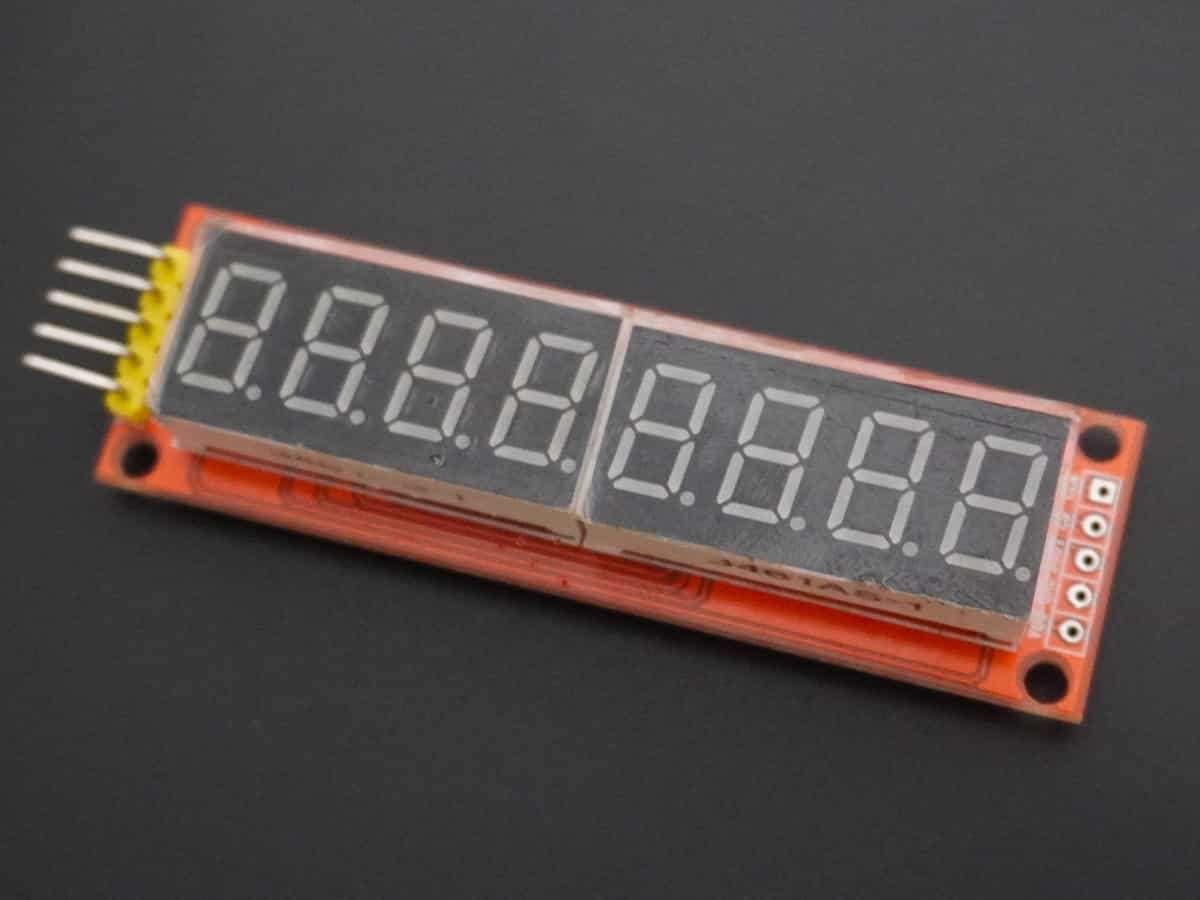
.jpg)
There are various display modules available in the market that make use of ICs like the MAX7219 to drive multiple 7-segment displays using SPI communication. One such module is shown in the image given below.
These modules are compact and require few pins and wires compared to using singular 7-segment displays in cascade.
MAX7219 7-Segment Display Hardware Connection with ESP32
.jpg)
.jpg)
Display 0 to 7 Digit on 7 Segment display
Here we have used the LedController library.
Download the library from the below link.
https://www.arduino.cc/reference/en/libraries/ledcontroller/
Code for MAX7219 with ESP32
/**
* @file LCDemo7Segment.ino
* @author Noa Sakurajin ([email protected])
* @brief using the ledcontroller with 7-segment displays
* @version 0.1
* @date 2020-12-30
*
* @copyright Copyright (c) 2020
*
*/
#include "LedController.hpp"
/*
You might need to change the following 3 Variables depending on your board.
pin 15 is connected to the DataIn
pin 14 is connected to the CLK
pin 13 is connected to LOAD/ChipSelect
*/
#define DIN 15
#define CS 13
#define CLK 14
/*
Now we need a LedController Variable to work with.
We have only a single MAX72XX so the Dimensions are 1,1.
*/
LedController<1,1> lc;
/* we always wait a bit between updates of the display */
unsigned long delaytime=1000;
void setup() {
//Here a new LedController object is created without hardware SPI.
lc=LedController<1,1>(DIN,CLK,CS);
lc.setIntensity(8); /* Set the brightness to a medium values */
lc.clearMatrix(); /* and clear the display */
}
void loop() {
for(int i=0; i<8; i++) {
lc.setDigit(0,i,i,false);
delay(delaytime);
}
lc.clearMatrix();
delay(delaytime);
}
- Now upload the code. (While uploading the code make sure your ESP32 board is in the boot mode.)
Output
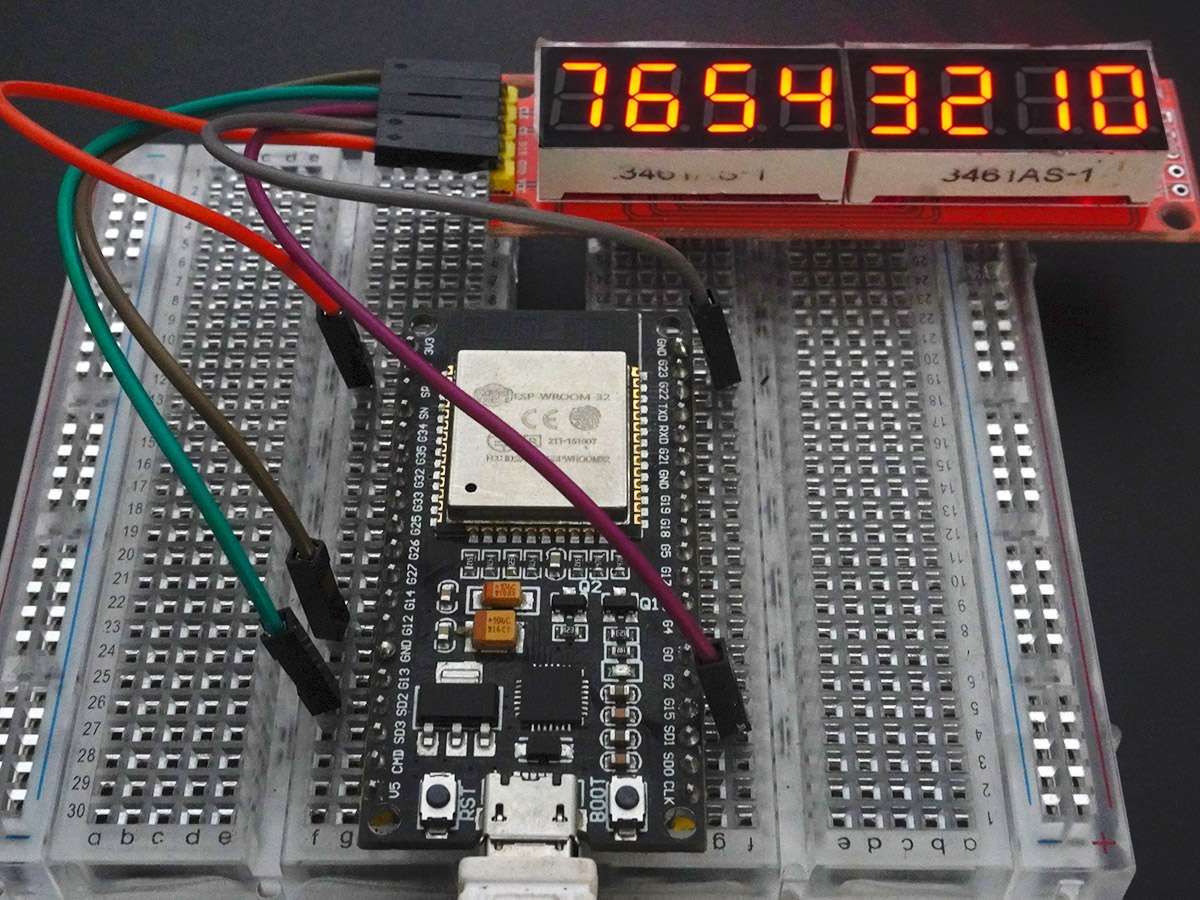
Let’s Understand the code
Set the SPI pin number
#define DIN 15
#define CS 13
#define CLK 14
Now we need a LedController
Variable to work with.
We have only a single MAX72XX so the Dimensions are 1,1.
LedController<1,1> lc;
we always wait a bit between updates of the display
unsigned long delaytime=1000;
In setup function
Here we have created a new LedController
object.
lc=LedController<1,1>(DIN,CLK,CS);
Set the display brightness to medium values
lc.setIntensity(8);
Clear the display
lc.clearMatrix();
In loop function
Set the digit and show it on the 7-segment display
for(int i=0; i<8; i++) {
lc.setDigit(0,i,i,false);
delay(delaytime);
}
Here wait for the delay time
delay(delaytime);
Components Used |
||
---|---|---|
MAX7456 On Screen Display Generator MAX7456 is a single channel monochrome on-screen display. It can be used for overlaying the text and/or graphics with SPI interface. |
X 1 | |
ESP32 WROOM WiFi Development Tools - 802.11 ESP32 General Development Kit, embeds ESP32-WROOM-32E, 4MB flash. |
X 1 |
Downloads |
||
---|---|---|
|
MAX7219_ESP32 | Download |