Overview of DHT11
- DHT11 is a single wire digital humidity and temperature sensor, which provides humidity and temperature values serially.
- It can measure the relative humidity in percentage (20 to 90% RH) and temperature in degree Celsius in the range of 0 to 50°C.
- It has 4 pins of which 2 pins are used for supply, 1 is not used and the last one is used for data.
- The data is the only pin used for communication. Pulses of different TON and TOFF are decoded as logic 1 or logic 0 or start pulse or end of a frame.
- For more information about the DHT11 sensor and how to use it, refer to the topic DHT11 sensor in the sensors and modules topic.
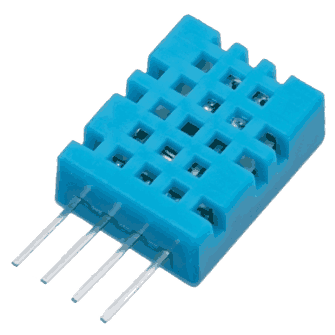
Connection Diagram of DHT11 to PIC18F4550
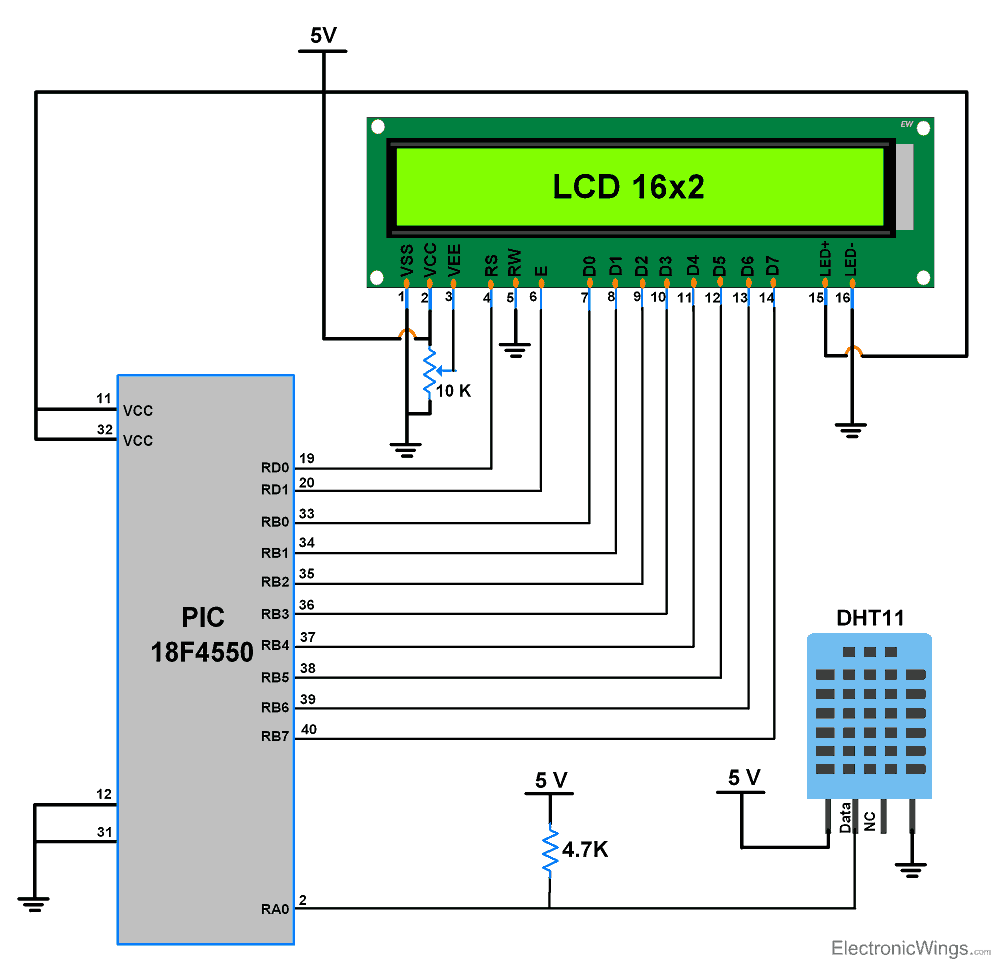
- The above circuit diagram shows the interfacing of PIC18F4550 with the DHT11 sensor.
- In that, a DHT11 sensor is connected to RA0 (PORTA).
Programming Steps
- First, initialize the LCD16x2_8-bit library.
- Define pin no. to interface DHT11 sensor, in our program we define RA0 (Pin no.2)
- Send the start pulse to the DHT11 sensor by making low to high on the data pin.
- Receive the response pulse from the DHT11 sensor.
- After receiving the response, receive 40-bit data serially from the DHT11 sensor.
- Display this received data on LCD16x2 along with error indication.
DHT11 Code for PIC18F4550
/*
* DHT11 Interfacing with PIC18F4550
* http://www.electronicwings.com
*/
#include <pic18f4550.h>
#include <xc.h>
#include <stdio.h>
#include "Configuration_Header_File.h"
#include "LCD_16x2_8-bit_Header_File.h"
#define Data_Out LATA0 /* assign Port pin for data*/
#define Data_In PORTAbits.RA0 /* read data from Port pin*/
#define Data_Dir TRISAbits.RA0 /* Port direction */
#define _XTAL_FREQ 8000000 /* define _XTAL_FREQ for using internal delay */
void DHT11_Start();
void DHT11_CheckResponse();
char DHT11_ReadData();
void main()
{
char RH_Decimal,RH_Integral,T_Decimal,T_Integral;
char Checksum;
char value[10];
OSCCON = 0x72; /* set internal oscillator with frequency 8 MHz*/
LCD_Init(); /* initialize LCD16x2 */
ADCON1=0x0F; /* this makes all pins as a digital I/O pins */
while(1)
{
DHT11_Start(); /* send start pulse to DHT11 module */
DHT11_CheckResponse(); /* wait for response from DHT11 module */
/* read 40-bit data from DHT11 module */
RH_Integral = DHT11_ReadData(); /* read Relative Humidity's integral value */
RH_Decimal = DHT11_ReadData(); /* read Relative Humidity's decimal value */
T_Integral = DHT11_ReadData(); /* read Temperature's integral value */
T_Decimal = DHT11_ReadData(); /* read Relative Temperature's decimal value */
Checksum = DHT11_ReadData(); /* read 8-bit checksum value */
/* convert humidity value to ascii and send it to display*/
sprintf(value,"%d",RH_Integral);
LCD_String_xy(0,0,value);
sprintf(value,".%d ",RH_Decimal);
LCD_String(value);
LCD_Char('%');
/* convert temperature value to ascii and send it to display*/
sprintf(value,"%d",T_Integral);
LCD_String_xy(1,0,value);
sprintf(value,".%d",T_Decimal);
LCD_String(value);
LCD_Char(0xdf);
LCD_Char('C');
sprintf(value,"%d ",Checksum);
LCD_String_xy(1,8,value);
/* check addition of humidity and temperature value equals to checksum */
if(Checksum != (RH_Integral + RH_Decimal + T_Integral + T_Decimal))
LCD_String_xy(0,8,"Error");
else
LCD_String_xy(0,8,"No Error");
MSdelay(500);
}
}
char DHT11_ReadData()
{
char i,data = 0;
for(i=0;i<8;i++)
{
while(!(Data_In & 1)); /* wait till 0 pulse, this is start of data pulse */
__delay_us(30);
if(Data_In & 1) /* check whether data is 1 or 0 */
data = ((data<<1) | 1);
else
data = (data<<1);
while(Data_In & 1);
}
return data;
}
void DHT11_Start()
{
Data_Dir = 0; /* set as output port */
Data_Out = 0; /* send low pulse of min. 18 ms width */
__delay_ms(18);
Data_Out = 1; /* pull data bus high */
__delay_us(20);
Data_Dir = 1; /* set as input port */
}
void DHT11_CheckResponse()
{
while(Data_In & 1); /* wait till bus is High */
while(!(Data_In & 1)); /* wait till bus is Low */
while(Data_In & 1); /* wait till bus is High */
}
Video of Measuring Temperature and Humidity using DHT11 and PIC18F4550
Components Used |
||
---|---|---|
DHT11 DHT11 is a single wire digital humidity and temperature sensor, which gives relative humidity in percentage and temperature in degree Celsius. |
X 1 | |
LCD16x2 Display LCD16x2 Display |
X 1 | |
PICKit 4 MPLAB PICKit 4 MPLAB |
X 1 | |
PIC18f4550 PIC18f4550 |
X 1 | |
Breadboard Breadboard |
X 1 |