Overview of NTC Thermistor
- A thermistor is a variable resistance element, whose resistance changes with the change in temperature.
- The resistance increases with an increase in temperature for PTC (Positive Temperature Coefficient) type thermistors.
- The resistance decreases with an increase in temperature for NTC (Negative Temperature Coefficient) type thermistors.
- They can be used as current limiters, temperature sensors, overcurrent protectors, etc.
- The change in resistance value is a measure of the temperature.
- By using the thermistor in series with a fixed resistance forming a simple voltage divider network, we can find out the temperature by the change in voltage of the voltage divider network due to a change in temperature. (Temperature change leads to change in resistance which leads to change in voltage.)
For more information about the thermistor and how to use it, refer to the topic NTC Thermistor in the sensors and modules section.
For information about ADC in PIC18F4550 and how to use it, refer to the topic ADC in PIC18F4550 in the PIC Inside section.
Connection Diagram of NTC Thermistor with PIC18F4550
Here, we used a 10k NTC thermistor.
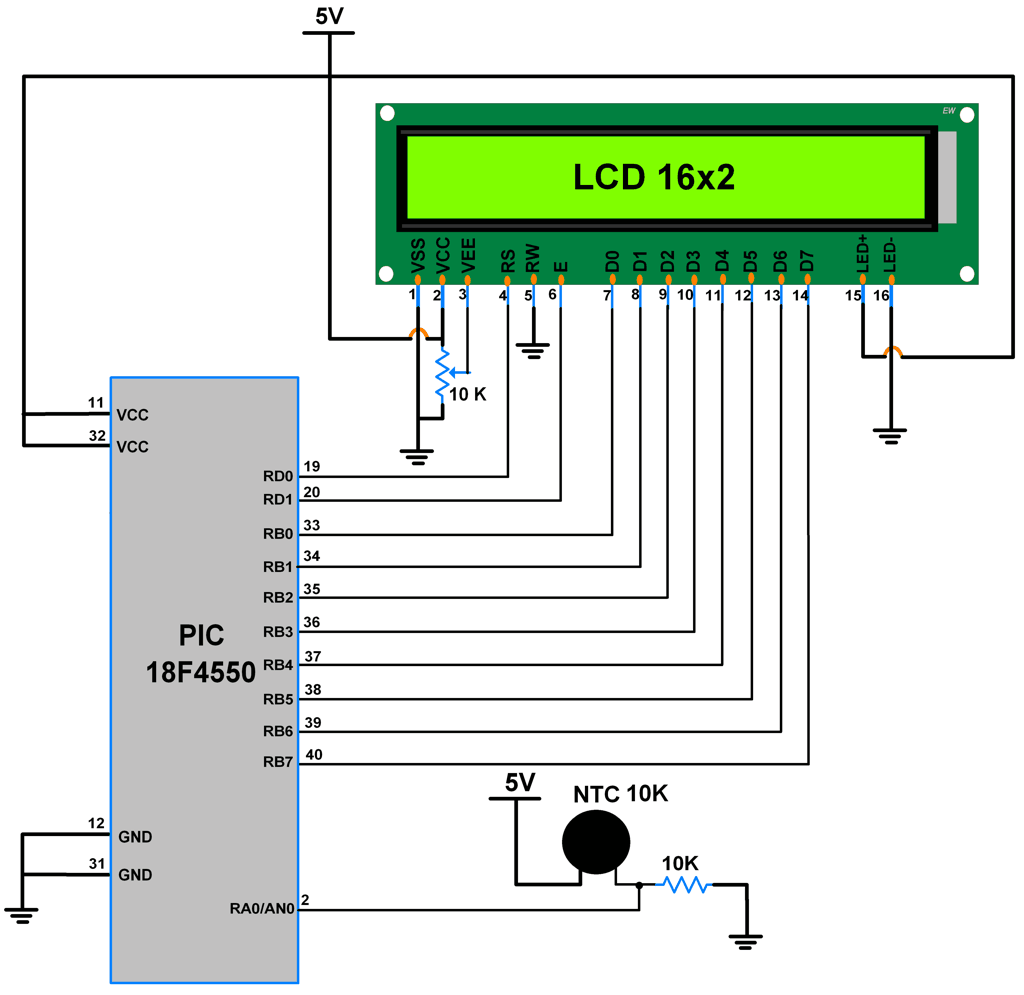
NTC Thermistor Code for PIC18F4550
/*
Thermistor Interfacing with PIC18F4550
http://www.electronicwings.com
*/
#include <pic18f4550.h>
#include <string.h>
#include <stdio.h>
#include "LCD_16x2_8-bit_Header_File.h"
#include "PIC18F4550_ADC_Header_File.h"
#include <math.h>
#define ohm 0xf4
#define B_coefficient 3950.00 /* B coefficient of NTC Thermistor*/
#define Room_temperature 25.00
#define Series_Resistance 10000.00
float Get_Temperature(int);
long NTC_Resistance;
void main(void)
{
float Temperature;
OSCCON =0x72;
LCD_Init(); /* initialize LCD16x2 */
LCD_Clear(); /* clear LCD */
ADC_Init(); /* initialize ADC */
char Temperature_buffer[20],Resistance[20];
int Analog_Input;
while(1)
{
Analog_Input = ADC_Read(0); /* store the analog data on a variable */
Temperature = Get_Temperature(Analog_Input);
sprintf(Temperature_buffer,"Temp: %.2f%cC ",Temperature,0xdf); /* convert integer to ASCII string */
LCD_String_xy(0, 0, Temperature_buffer);
sprintf(Resistance,"Res: %ld %c ",NTC_Resistance,ohm);
LCD_String_xy(1,0,Resistance);
MSdelay(1000); /* wait for 1 second */
}
}
float Get_Temperature(int analog_value)
{
float Thermistor_temperature;
analog_value = ADC_Read(0); /* store adc value on val register */
/* calculate the NTC resistance */
NTC_Resistance = ((1023*Series_Resistance/analog_value) - Series_Resistance);
Thermistor_temperature = log(NTC_Resistance); /* calculate natural log of resistance */
/* Calculate Temperature using B parameter Equation */
/* 1/T = 1/T0 + ((1/B_coefficient)*log(NTC_Resistance/Series_Resistance)) */
Thermistor_temperature = (1.0/(Room_temperature + 273.15))+
(1.0/B_coefficient)*log(NTC_Resistance/Series_Resistance));
Thermistor_temperature = (1/Thermistor_temperature) - 273.15; /* convert kelvin to °C */
return Thermistor_temperature;
}
Video of Temperature Measurement using NTC Thermistor and PIC18F4550
Components Used |
||
---|---|---|
NTC Thermistor NTC Thermistor |
X 1 | |
PIC18f4550 PIC18f4550 |
X 1 | |
LCD16x2 Display LCD16x2 Display |
X 1 |
Downloads |
||
---|---|---|
|
Thermistor_Interfacing_PIC18F4550_Project_File | Download |