Overview of Nokia5110
Nokia5110 is a graphical display that can display text, images, and various patterns.
It has a resolution of 48x84 and comes with a backlight.
It uses SPI communication to communicate with a microcontroller.
Data and commands can be sent through a microcontroller to the display to control the display output.
It has 8 pins.
For more information about the Nokia5110 display and how to use it, refer to the topic Nokia5110 Graphical Display in the sensors and modules section.
For information on SPI in PIC18F4550, refer to the topic on SPI in PIC18F4550 in the PIC inside section.
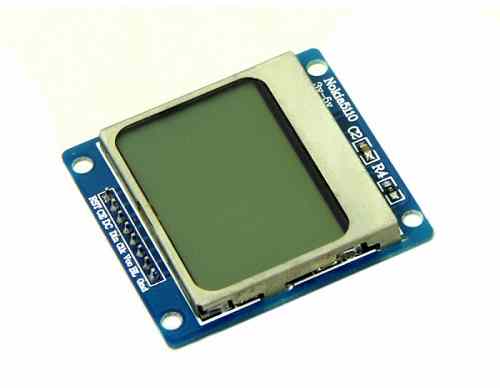
Connection Diagram for Nokia 5110 Display with PIC18F4550
- The following circuit diagram shows the complete interfacing of PIC18F4550 to the Nokia5110 display.
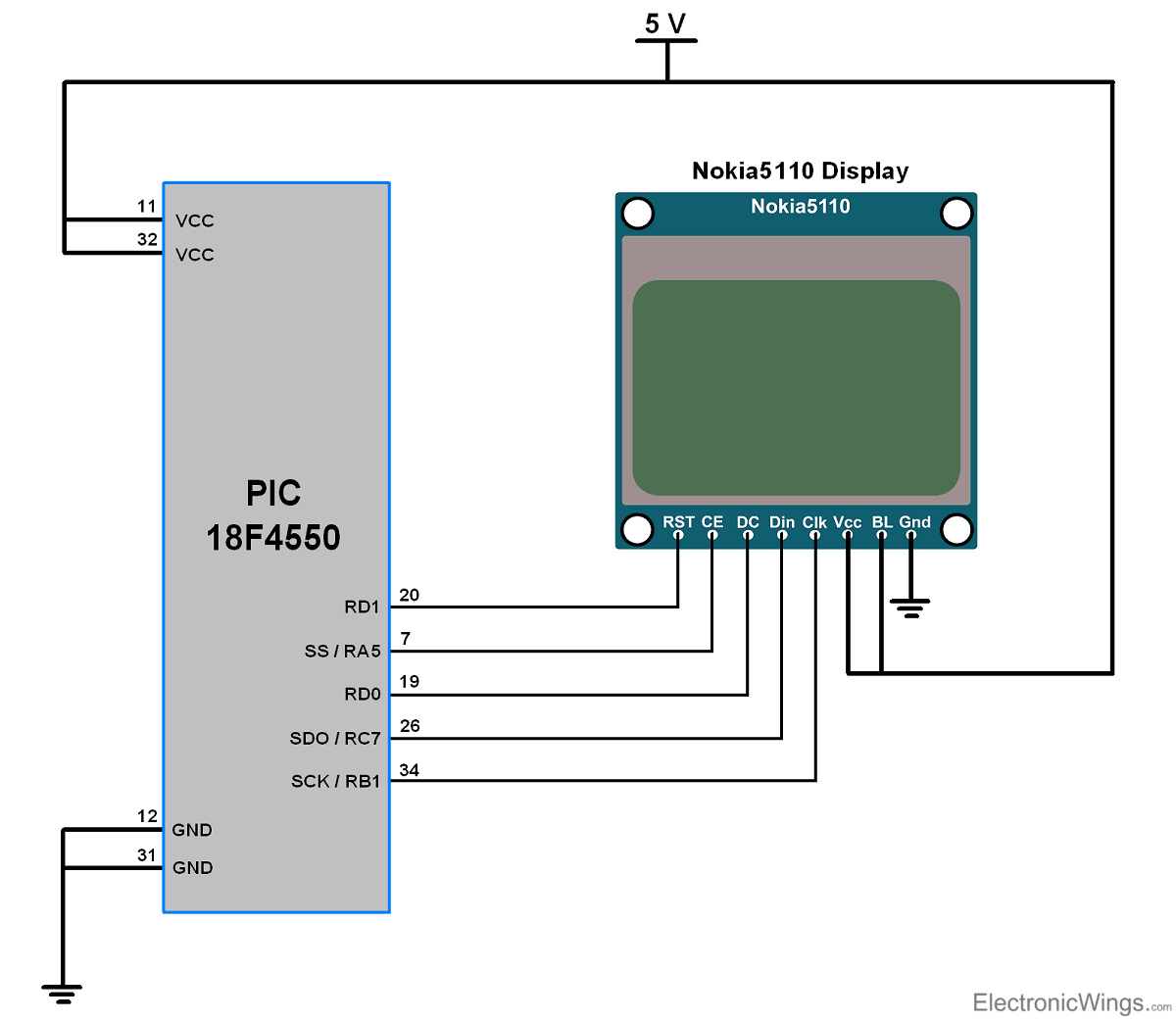
Interconnection Detail
Nokia5110 Pins | PIC18F4550 Pins | PIC18F4550 Ports/Function |
---|---|---|
RST | 20 | RD1 |
CE | 7 | SS |
DC | 19 | RD0 |
Din | 26 | SDO |
CLK | 34 | SCK |
BL and VCC | 11/32 | VCC |
GND | 12/31 | GND |
Programming of Nokia5110 Display
- In the coding first step is to include and initialize the SPI_Header_File.h header files.
- In the SPI_Header_File.h file contains all definitions of SPI.
Initialization of Nokia5110
- The first step is to reset the display by sending low to high pulse to the reset pin. The pulse width of a low signal should not be < 100 ms.
- Send command 0x21 to set the display in extended command mode (H=1).
- Set the voltage bias system using a 0x13 command, it is recommended for n=4 and 1:48 mux rate.
- Set the temp. coefficient. E.g. Set temperature coefficient 3 by sending 0x07H.
- Then set VOP = 5 V by sending command 0xC0H.
- Send the command 0x20 to configure the display for basic command mode (H=0).
- And then send 0x0C to operate the display in normal mode.
void Nokia_Init()
{
/*apply 100 ms reset(low to high) pulse */
RES = 0; /* enable reset */
MSdelay(100);
RES = 1; /* disable reset */
Nokia_SendCommand(0x21); /* use extended instruction set */
Nokia_SendCommand(0x13); /* select Bias voltage */
Nokia_SendCommand(0x07); /* set temperature coefficient */
Nokia_SendCommand(0xC0); /* set LCD Vop for contrast */
Nokia_SendCommand(0x20); /* use basic instruction set */
Nokia_SendCommand(0x0C); /* set normal mode */
}
Command write the function:
- Make DC pin low for command operation.
- Enable the slave select pin.
- Send/write the command to the SPI data(SSPBUF) register.
- Disable the slave select pin.
void Nokia_SendCommand(char cmd)
{
DC = 0; /* Data/Command pin, D/C=1 - Data, D/C = 0 - Command*/
CS = 0; /* enable chip */
SPI_Write (cmd); /* write command to the Nokia 5110 */
CS = 1; /* disable chip */
}
Data write the function:
- For data, the operation makes DC pin high for data operation.
- Enable the slave select pin.
- Send/write the data to the SPI data(SSPBUF) register.
- Disable the slave select pin after sending the data.
void Nokia_SendData(char dat)
{
char i;
DC = 1; /* Data/Command pin, D/C=1 - Data, D/C = 0 - Command*/
CS = 0; /* enable chip */
for(i=0;i<5;i++)
SPI_Write(font[(dat) - (0x20)][i]); /* write data to the Nokia 5110 */
CS = 1; /* disable chip */
}
Nokia 5110 Display Code for PIC18F4550
Display “ElectronicWings” on Nokia5110 display using PIC18F4550
/*
* Nokia 5110 display interface with PIC18F4550
* http://www.electronicwings.com
*
*/
#include <pic18f4550.h>
#include "Configuration_Header_File.h"
#include "SPI_Header_File.h"
#include "Nokia_Font.h"
void Nokia_Init();
void Nokia_SendCommand(char);
void Nokia_SendData(char);
void Nokia_SendString(char *);
void MSdelay(unsigned int);
void Nokia_Clear();
void Nokia_PositionXY(char, char);
#define DC LATD0
#define RES LATD1 /* connected to reset */
void main()
{
OSCCON = 0X72; /* set internal oscillator frequency, 8 MHz*/
TRISD = 0; /* set PORT as output port*/
SPI_Init_Master(); /* initialize SPI master*/
Nokia_Init(); /* initialize Nokia 5110 display */
Nokia_Clear(); /* clear Nokia display */
Nokia_PositionXY(0,0); /* set X and Y position for printing */
Nokia_SendString("ElectronicWings");
while(1);
}
void Nokia_SendCommand(char cmd)
{
DC = 0; /* Data/Command pin, D/C=1 - Data, D/C = 0 - Command*/
CS = 0; /* enable chip */
SPI_Write(cmd); /* write command to the Nokia 5110 */
CS = 1; /* disable chip */
}
void Nokia_SendData(char dat)
{
char i;
DC = 1; /* Data/Command pin, D/C=1 - Data, D/C = 0 - Command*/
CS = 0; /* enable chip */
for(i=0;i<5;i++)
SPI_Write(font[(dat) - (0x20)][i]); /* write data to the Nokia 5110 */
CS = 1; /* disable chip */
}
void Nokia_SendString(char *data)
{
char i;
while((*data)!=0)
{
Nokia_SendData(*data);
data++;
}
}
void Nokia_PositionXY(char X, char Y)
{
Nokia_SendCommand(0x80 | X); /* set X position */
Nokia_SendCommand(0x40 | Y); /* set Y position */
}
void Nokia_Init()
{
/*apply 100 ms reset(low to high) pulse */
RES = 0; /* enable reset */
MSdelay(100);
RES = 1; /* disable reset */
Nokia_SendCommand(0x21); /* display extended commands*/
Nokia_SendCommand(0x13); /* select Bias voltage*/
Nokia_SendCommand(0x07); /* set temperature coefficient*/
Nokia_SendCommand(0xC0); /* set LCD Vop for contrast */
Nokia_SendCommand(0x20); /* display basic commands */
Nokia_SendCommand(0x0C); /* set normal mode */
}
void Nokia_Clear()
{
char i,j;
CS = 0;
DC = 1;
for(i=0;i<6;i++)
{
for(j=0;j<84;j++)
SPI_Write(0); /*write 0 to clear display */
}
CS = 1;
}
void MSdelay(unsigned int val)
{
unsigned int i,j;
for(i=0;i<=val;i++)
for(j=0;j<81;j++); /*This count Provide delay of 1 ms for 8MHz Frequency */
}
Image Display Function for Nokia5110
void Nokia_DisplayImage (const unsigned char *image)
{
int i;
CS = 0;
DC = 1;
for(i=0;i<504;i++)
{
SPI_Write(image[i]);
}
CS = 1;
}
Video of Nokia5110 Display using PIC18F4550
Components Used |
||
---|---|---|
Nokia5110 Graphical Display Nokia5110 is 48x84 dot LCD display with Serial Peripheral Interface (SPI) Connectivity. It was designed for cell phones. It is also used in embedded applications. |
X 1 | |
PIC18f4550 PIC18f4550 |
X 1 | |
PICKit 4 MPLAB PICKit 4 MPLAB |
X 1 |